
Computers and Technology, 24.06.2021 20:40 kirstennnash
Event Handlers
Return to the mt_calc. js file in your editor. Directly below the initial comment section, insert a command that runs the init() function when the page is loaded by the browser.
Create the init() function, which sets up the event handlers for the page. Within the init()function, add the following commands:
Declare the calcButtons variable containing the collection of page elements belonging to the calcButton class.
Loop through the calcButtons object collection and, for each button in that collection, run the buttonClick() function in response to the click event.
After the for loop, add a command that runs the calcKeys() function in response to the keydown event occurring within the element with the ID "calcWindow".
JavaScript Functions
Create the buttonClick() function. The purpose of this function is to change what appears in the calculator window when the user clicks the calculator buttons. Add the following commands to the function:
Declare the calcValue variable equal to the value attribute of the calcWindow text area box.
Declare the calcDecimal variable equal to the value attribute of the decimals input box.
Each calculator button has a valueattribute that defines what should be done with that button. Declare the buttonValueattribute equal to the value attribute of the event object target.
Create a switch-case structure for the following possible values of the buttonValue variable:
For "del", delete the contents of the calculate window by changing calcValue to an empty text string.
For "bksp", erase the last character in the calculator window by changing calcValue to the value returned by the eraseChar() function using calcValueas the parameter value.
For "enter", calculate the value of the current expression by changing calcValue to: " = " + evalEq(calcValue, calcDecimal) + "\n"Note that \n is used to add a line return at the end of the answer.
For "prev", copy the last equation in the calculator window by changing calcValue to the value returned by the lastEq() function using calcValue as the parameter value.
Otherwise, append the calculator button character to the calculator window by letting, calcValue equal calcValueplus buttonValue.
After the switch-case structure, set the value attribute of the calcWindow text area box to calcValue.
Run the command document. getElementById("calcWindow").focus( )to put the cursor focus within the calculator window.
Next, you will control the keyboard actions within the calculator window. Theresa wants you to program the actions that will happen when the user presses the Delete, Enter, and up-arrow keys. Add the calcKeys() function containing the following commands:
As you did in the buttonClick() function, declare the calcValue and calcDecimalvariables.
Create a switch-case structure for different values of the key attribute of the event object as follows:
For "Delete", erase the contents of the calculator window by changing calcValue to an empty text string.
For "Enter", add the following expression to calcValue:
" = " + evalEq(calcValue, calcDecimal)
For "ArrowUp", add the following expression to calcValue
lastEq(calcWindow. value)
And then enter a command that prevents the browser from performing the default action in response to the user pressing the up-arrow key.
After the switch-case structure, set the value attribute of the calcWindow text area box to calcValue.
Here's the code:
"use strict";
/*
New Perspectives on HTML5, CSS3 and JavaScript 6th Edition
Tutorial 11
Case Problem
Author: DD
Date: 04/18/19
Filename: mt_calc. js
Functions List:
init()
Initializes the contents of the web page and sets up the
event handlers
buttonClick(e)
Adds functions to the buttons clicked within the calcutlor
calcKeys(e)
Adds functions to key pressed within the calculator window
eraseChar(textStr)
Erases the last character from the text string, textStr
evalEq(textStr, decimals)
Evaluates the equation in textStr, returning a value to the number of decimals specified by the decimals parameter
lastEq(textStr)
Returns the previous expression from the list of expressions in the textStr parameter
*/
window. onload = init;
function init() {
var calButtons =
}
function buttonClick(e) {
}
function calcKeys(e) {
}
/* */
function eraseChar(textStr) {
return textStr. substr(0, textStr. length - 1);
}
function evalEq(textStr, decimals) {
var lines = textStr. split(/\r?\n/);
var lastLine = lines[lines. length-1];
var eqValue = eval(lastLine);
return eqValue. toFixed(decimals);
}
function lastEq(textStr) {
var lines = textStr. split(/\r?\n/);
var lastExp = lines[lines. length-2];
return lastExp. substr(0, lastExp. indexOf("=")).trim();
}

Answers: 2
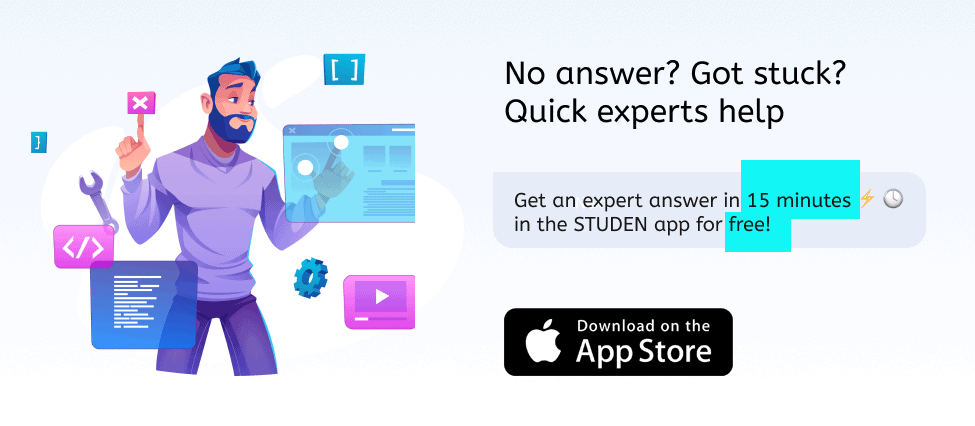
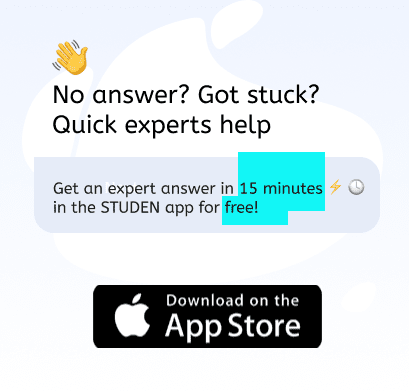
Another question on Computers and Technology

Computers and Technology, 21.06.2019 20:20
Sometimes writers elaborate on the truth when recalling past events so they can enhance their narrative essay with more interesting descriptions. do you feel that published writers should or should not embellish real life events just to make their stories more interesting?
Answers: 2

Computers and Technology, 21.06.2019 22:00
Formula that contains a nested function that first calculates the average of the values in range e6: p6 and uses the round function to round that average to the nearest 10 dollars. use -1 for the value of the number digit argument. would the formula be =round(average(e6: p6),0
Answers: 1

Computers and Technology, 22.06.2019 17:40
Gabe wants to move text from one document to another document. he should copy the text, paste the text, and open the new document highlight the text, select the cut command, move to the new document, make sure the cursor is in the correct location, and select the paste command select the save as command, navigate to the new document, and click save highlight the text, open the new document, and press ctrl and v
Answers: 1

Computers and Technology, 23.06.2019 01:30
Negative methods of behavior correction include all but this: sarcasm verbal abuse setting an example for proper behavior humiliation
Answers: 1
You know the right answer?
Event Handlers
Return to the mt_calc. js file in your editor. Directly below the initial comment se...
Questions

Biology, 29.01.2020 21:46
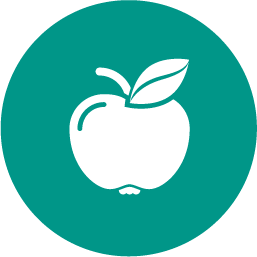
Physics, 29.01.2020 21:46



Biology, 29.01.2020 21:46
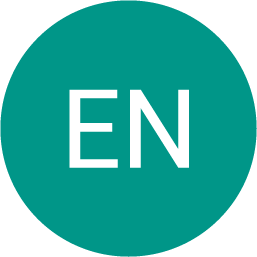
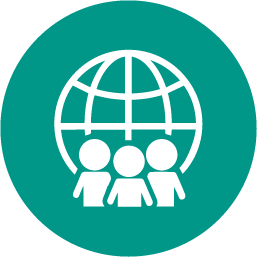




Mathematics, 29.01.2020 21:46
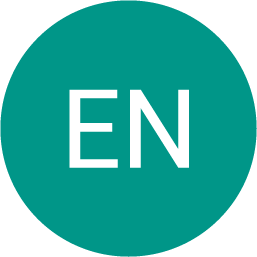
English, 29.01.2020 21:46



Chemistry, 29.01.2020 21:46



Business, 29.01.2020 21:46

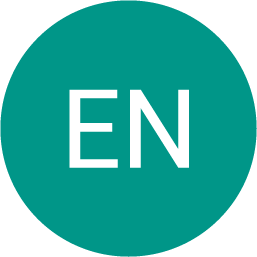
English, 29.01.2020 21:46