
Computers and Technology, 19.07.2021 21:20 snurpeisov
IN C++
A. Create an abstract base class called Currency with two integer attributes, both of which are non-public. The int attributes will represent whole part (or currency note value) and fractional part (or currency coin value) such that 100 fractional parts equals 1 whole part.
B. Create one derived class - Money - with two additional non-public string attributes which will contain the name of the currency note (Dollar) and currency coin (Cent) respectively. DO NOT add these attributes to the base Currency class.
C. In your base Currency class, add public class (C++ students are allowed to use friend methods as long as a corresponding class method is defined as well) methods for the following, where appropriate:
Default Construction (i. e. no parameters passed)
Construction based on parameters for all attributes - create logical objects only, i. e. no negative value objects allowed
Copy Constructor and/or Assignment, as applicable to your programming language of choice
Destructor, as applicable to your programming language of choice
Setters and Getters for all attributes
Adding two objects of the same currency
Subtracting one object from another object of the same currency - the result should be logical, i. e. negative results are not allowed
Comparing two objects of the same currency for equality/inequality
Comparing two objects of the same currency to identify which object is larger or smaller
Print method to print details of a currency object
All of the above should be instance methods and not static.
The add and subtract as specified should manipulate the object on which they are invoked. It is allowed to have overloaded methods that create ane return new objects.
D. In your derived Money class, add new methods or override inherited methods as necessary, taking care that code should not be duplicated or duplication minimized. Think modular and reusable code.
E. Remember -
Do not define methods that take any decimal values as input in either the base or derived classes.
Only the print method(s) in the classes should print anything to console.
Throw String (or equivalent) exceptions from within the classes to ensure that invalid objects cannot be created.
F. In your main:
Declare a primitive array of 5 Currency references (for C++ programmers, array of 5 Currency pointers).
Ask the user for 5 decimal numbers to be input - for each of the inputs you will create one Money object to be stored in the array.
Once the array is filled, perform the following five operations:
Print the contents of the array of objects created in long form, i. e. if the user entered "2.85" for the first value, it should be printed as "2 Dollar 85 Cent".
Add the first Money object to the second and print the resulting value in long form as above.
Subtract the first Money object from the third and print the resulting value in long form as above.
Compare the first Money object to the fourth and print whether both objects are equal or not using long form for object values.
Compare the first Money object to the fifth and print which object value is greater than the other object value in long form.
All operations in the main should be performed on Currency objects demonstrating polymorphism.
Remember to handle exceptions appropriately.
There is no sample output - you are allowed to provide user interactivity as you see fit.

Answers: 2
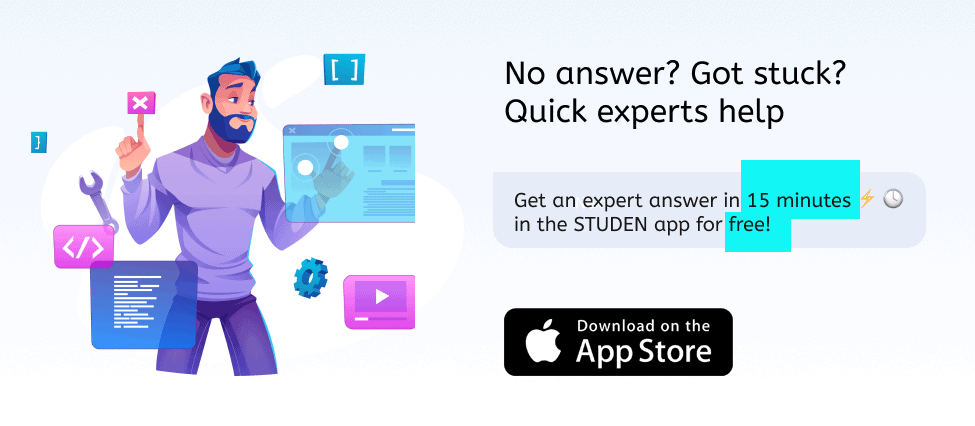
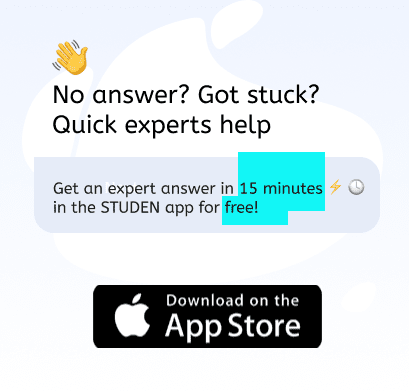
Another question on Computers and Technology

Computers and Technology, 22.06.2019 10:30
Choose the best explanation for the following statement communication is symbolic
Answers: 3

Computers and Technology, 22.06.2019 23:00
Suppose s, t, and w are strings that have already been created inside main. write a statement or statements, to be added to main, that will determine if the lengths of the three strings are in order by length, smallest to largest. that is, your code should determine if s is strictly shorter than t, and if t is strictly shorter than w. if these conditions hold your code should print (the boolean value) true. if not, your code should print false. (strictly means: no ties) example: if s, t, and w are "cat", "hats", and "skies" your code should print true - their lengths are 3-4-5; but if s, t, and w are "cats" "shirt", and "trust", then print false - their lengths are 4-5-5 enter your code in the box below
Answers: 2

Computers and Technology, 23.06.2019 07:30
What is the original authority for copyright laws
Answers: 1

Computers and Technology, 24.06.2019 17:40
File i/o activity objective: the objective of this activity is to practice working with text files in c#. for this activity, you may do all code in the main class. instructions: create an app that will read integers from an input file name numbers.txt that will consist of one integer per record. example: 4 8 25 101 determine which numbers are even and which are odd. write the even numbers to a file named even.txt and the odd numbers to a file named odd.txt.
Answers: 3
You know the right answer?
IN C++
A. Create an abstract base class called Currency with two integer attributes, both of which...
Questions
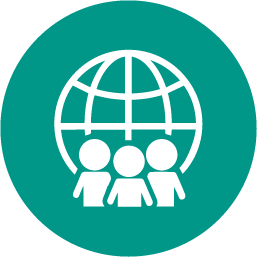

Biology, 25.01.2020 12:31
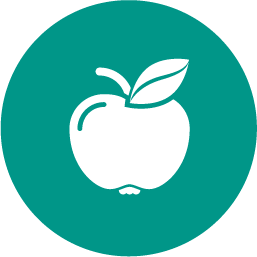

Mathematics, 25.01.2020 12:31


Mathematics, 25.01.2020 12:31

Mathematics, 25.01.2020 12:31

Geography, 25.01.2020 12:31

SAT, 25.01.2020 12:31
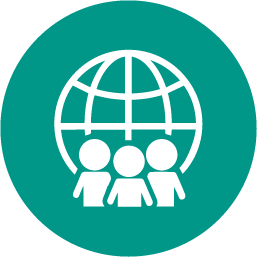
History, 25.01.2020 12:31

Business, 25.01.2020 12:31


Chemistry, 25.01.2020 12:31

Mathematics, 25.01.2020 12:31

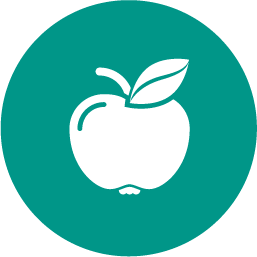


Chemistry, 25.01.2020 12:31
