
Computers and Technology, 20.08.2021 01:00 mikaelalcool49
Here is the starting code for your assignment. As you can see, so far there is a function to create two random numbers between 1 and 25 and a function to add them together. Your assignment is to create three more functions, add the prototypes correctly, call them from main correctly and output the results. The three functions should be subtractNumbers, multiplyNumbers, and divideNumbers. Remember that division won't always yield an integer so be sure to take care of that issue within the function (don't change the variable declarations).
Don't change the code that I've given you.
Follow the same pattern.
Don't use tools that we haven't studied.
// This program adds, subtracts, multiplies, and divides two random numbers
#include
#include // For rand and srand
#include // For the time function
using namespace std;
// Function declarations
int chooseNumber();
int addNumbers(int, int);
const int MIN_VALUE = 1; // Minimum value
const int MAX_VALUE = 25; // Maximum value
unsigned seed = time(0);
int main()
{
// Seed the random number generator.
srand(seed);
int firstNumber = chooseNumber();
int secondNumber = chooseNumber();
int sum = addNumbers(firstNumber, secondNumber);
cout << "The first number is " << firstNumber << endl;
cout << "The second number is " << secondNumber << endl;
cout << "The sum is " << sum;
return 0;
}
int chooseNumber()
{
// Variable
int number; // To hold the value of the number
number = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE;
return number;
}
int addNumbers (int number1, int number2)
{
int number = number1 +number2;
return number;
}
Notice that number is used as a variable in more than one function. This works because number is a variable.

Answers: 2
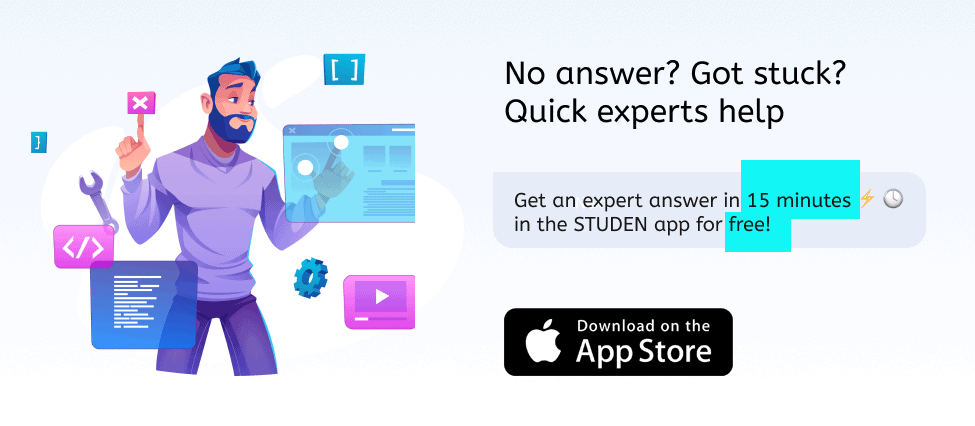
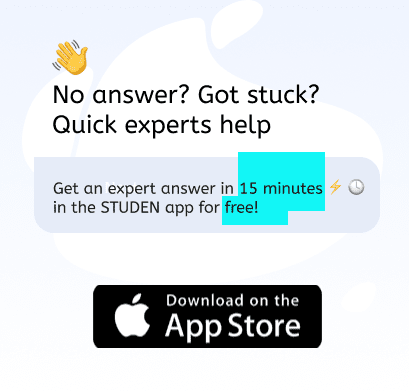
Another question on Computers and Technology

Computers and Technology, 21.06.2019 18:30
Which statement best explains how plant cells use photosynthesis to maintain homeostasis? they use glucose and water to make energy. they break down glucose into fat. they combine oxygen and water to make glucose. they make glucose from carbon dioxide and water.
Answers: 1

Computers and Technology, 23.06.2019 00:00
What engine component is shown in the above figure?
Answers: 1

Computers and Technology, 23.06.2019 06:30
Which option correctly describes a dbms application? a. software used to manage databases b. software used to organize files and folders c. software used to develop specialized images d. software used to create effective presentations
Answers: 1

Computers and Technology, 23.06.2019 06:30
On early television stations, what typically filled the screen from around 11pm until 6am? test dummies test patterns tests testing colors
Answers: 1
You know the right answer?
Here is the starting code for your assignment. As you can see, so far there is a function to create...
Questions


Mathematics, 06.05.2021 04:30
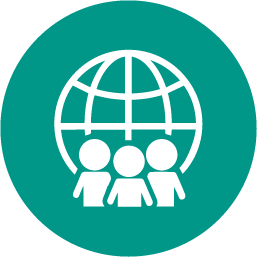


Mathematics, 06.05.2021 04:30
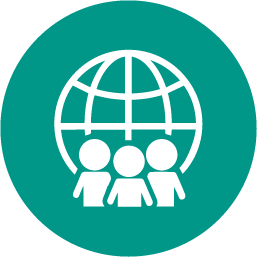
History, 06.05.2021 04:30

Chemistry, 06.05.2021 04:30

Mathematics, 06.05.2021 04:30


Mathematics, 06.05.2021 04:30
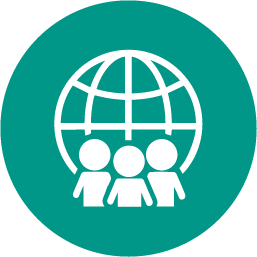

Mathematics, 06.05.2021 04:30


Mathematics, 06.05.2021 04:30

Engineering, 06.05.2021 04:30
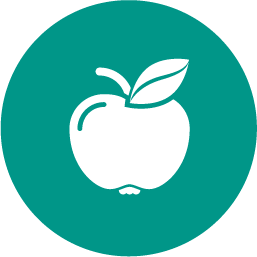

Chemistry, 06.05.2021 04:30

Mathematics, 06.05.2021 04:30

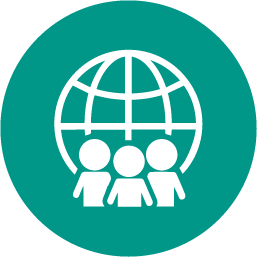
History, 06.05.2021 04:30