
Computers and Technology, 08.09.2021 19:50 glowbaby123
The function retrieveAt of the class arrayListType is written as a void function. Rewrite this function so that it is written as a value returning function, returning the required item. If the location of the item to be returned is out of range, use the assert function to terminate the program. Also, write a program to test your function. Use the class unorderedArrayListType to test your function.
we have arrayListType. h
#ifndef H_arrayListType
#define H_arrayListType
#include
#include
class arrayListType {
public:
bool isFull() const;
int listSize() const;
int maxListSize() const;
void print() const;
bool isItemAtEqual(int location, int item) const;
virtual void insertAt(int location, int insertItem) = 0;
virtual void insertEnd(int insertItem) = 0;
void removeAt(int location);
// void retrieveAt(int location, int& retItem) const;
int retrieveAt(int location, array){
if(location <= array. size() - 1){
return array[location]; } else{
cout<<"Location out of range";
assert(); // Assuming the assert function is defined in the program. }}
int arrayListType::retrieveAt(int location) const{
int retItem = 0;
assert( (location < 0) || (location >= length));
retItem = list[location];
return retItem;} //end retrieveAt*/
//int retrieveAt(int location) const;
virtual void replaceAt(int location, int repItem) = 0;
void clearList();
virtual int seqSearch(int searchItem) const = 0;
virtual void remove(int removeItem) = 0;
arrayListType (const arrayListType& otherList);
virtual ~arrayListType();
protected:
int *list; //array to hold the list elements
int length; //variable to store the length of the list
int maxSize; //variable to store the maximum
//size of the list
}; #endif
arrayListTypeImp. cpp
#include
#include
#include "arrayListType. h"
using namespace std;
bool arrayListType::isEmpty() const{
return (length == 0);
} //end isEmpty
bool arrayListType::isFull() const{
return (length == maxSize);
} //end isFull
int arrayListType::listSize() const{
return length;
} //end listSize
int arrayListType::maxListSize() const{
return maxSize;
} //end maxListSize
void arrayListType::print() const{
for (int i = 0; i < length; i++)
cout << list[i] << " ";
cout << endl;
} //end print
bool arrayListType::isItemAtEqual(int location, int item) const{
if (location < 0 || location >= length) {
cout << "The location of the item to be removed "
<< "is out of range." << endl;
return false;
}
else
return (list[location] == item);
} //end isItemAtEqual
void arrayListType::removeAt(int location){
if (location < 0 || location >= length)
cout << "The location of the item to be removed "
<< "is out of range." << endl;
else {
for (int i = location; i < length - 1; i++)
list[i] = list[i+1];
length--;
}
} //end removeAt
void arrayListType::retrieveAt(int location, int& retItem) const{
if (location < 0 || location >= length)
cout << "The location of the item to be retrieved is "
<< "out of range" << endl;
else
retItem = list[location];
} //end retrieveAt
void arrayListType::clearList(){
length = 0;
} //end clearList
arrayListType::arrayListType(int size){
if (size <= 0; {
cout << "The array size must be positive. Creating "
<< "an array of the size 100." << endl;
maxSize = 100;}
else
maxSize = size;
length = 0;
list = new int[maxSize];
} //end constructor
arrayListType::~arrayListType(){
delete [] list;
} //end destructor
arrayListType::arrayListType(const arrayListType& otherList){
maxSize = otherList. maxSize;
length = otherList. length;
list = new int[maxSize]; //create the array
for (int j = 0; j < length; j++) //copy otherList
list [j] = otherList. list[j];
}//end copy constructor

Answers: 1
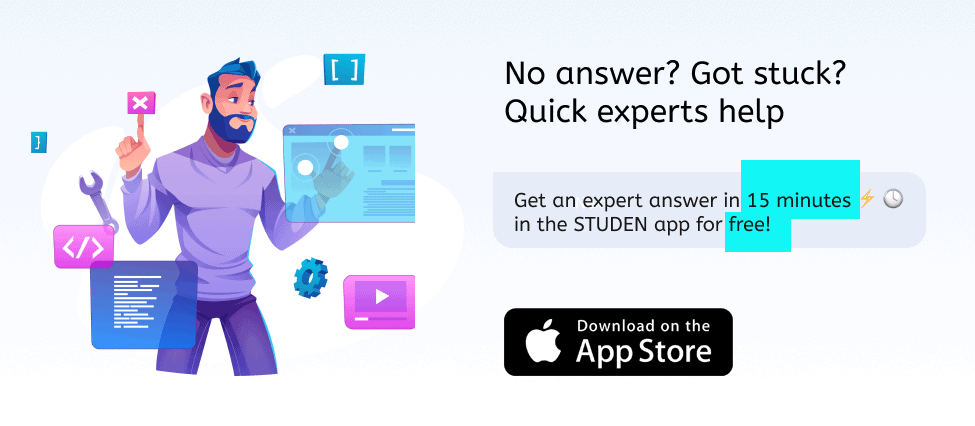
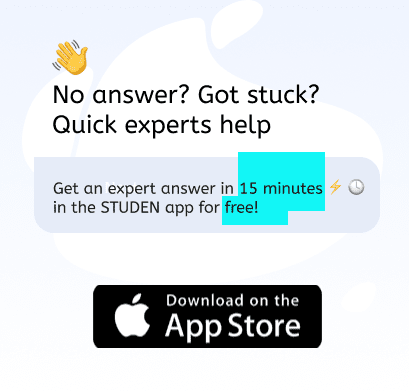
Another question on Computers and Technology

Computers and Technology, 23.06.2019 14:00
In which job role will you be creating e-papers, newsletters and preiodicals
Answers: 1

Computers and Technology, 24.06.2019 00:00
Consider the series where in this problem you must attempt to use the ratio test to decide whether the series converges. compute enter the numerical value of the limit l if it converges, inf if it diverges to infinity, minf if it diverges to negative infinity, or div if it diverges but not to infinity or negative infinity.
Answers: 1

Computers and Technology, 24.06.2019 00:00
Which tool could be used to display only rows containing presidents who served two terms
Answers: 3

Computers and Technology, 24.06.2019 13:30
What is the most important for you to choose before you build a network?
Answers: 1
You know the right answer?
The function retrieveAt of the class arrayListType is written as a void function. Rewrite this funct...
Questions



Mathematics, 24.05.2021 21:10

Mathematics, 24.05.2021 21:10
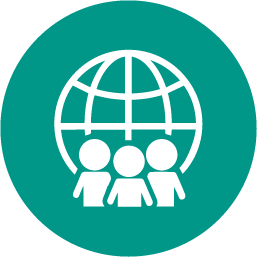
History, 24.05.2021 21:10

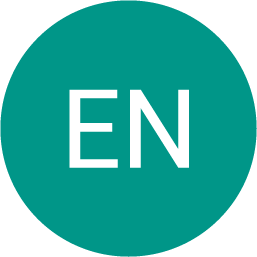

Mathematics, 24.05.2021 21:10
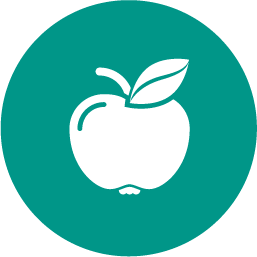

Mathematics, 24.05.2021 21:10

Mathematics, 24.05.2021 21:10

Mathematics, 24.05.2021 21:10

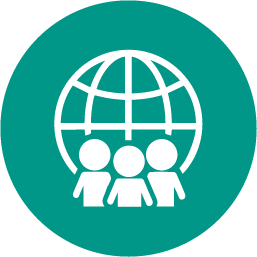
Social Studies, 24.05.2021 21:10


Mathematics, 24.05.2021 21:10


Mathematics, 24.05.2021 21:10
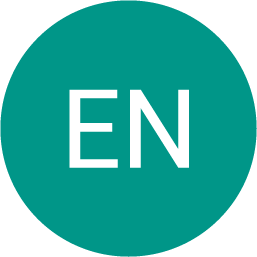