
Computers and Technology, 19.10.2021 02:20 neariah24
The C library function memset(p, c,n) writes (uint8_t)(c) repeatedly n times begininig at the address held in pointer p. Sometimes it is more efficient or convenient to copy a multibyte quantity instead of a single byte one. Implement the following function.
void memset16(void *b, int num_bytes, void *pattern16)
In this function, b has the address of a memory buffer that is num_bytes long. The function should repeatedly copy the 16 byte pattern that pattern16 points at into the memory buffer until num_bytes have been written. If num_bytes is not a multple of 16, the final wriite of the 16 byte pattern should be truncated to finish filling the buffer.
For example if the 16 bytes that pattern16 points at is 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff, then memset(b, 20, pattern16) should write to the buffer pointed at by p the 20 bytes 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff 00 11 22 33.
Use SSE instructions to improve efficiency. Here's pseudocode.
x = SSE unaligned load from pattern16
while (num_bytes >= 16)
SSE unaligned store x to p
advance p by 16 bytes
decrement num_bytes by 16
while (num_bytes > 0)
store 1 byte from pattern16 to p
advance p by 1 byte
advance pattern16 by 1 byte
decrement num_bytes by 1
void memset16(void *p, void *pattern16, int num_bytes)

Answers: 1
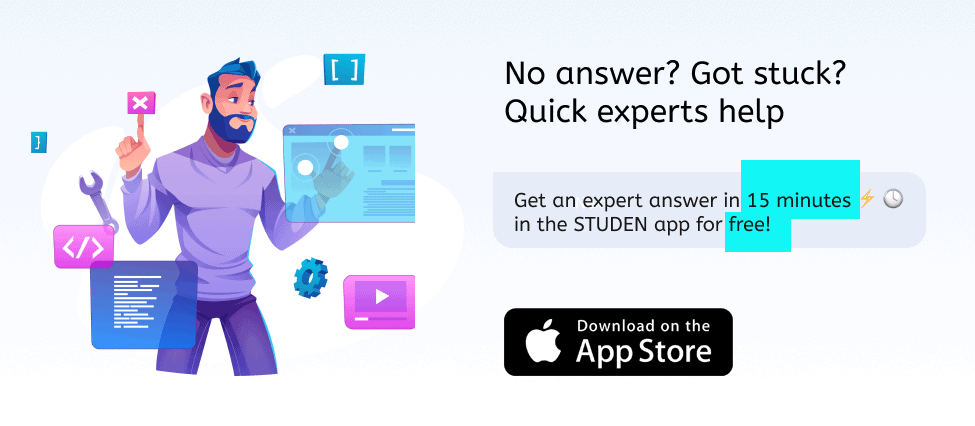
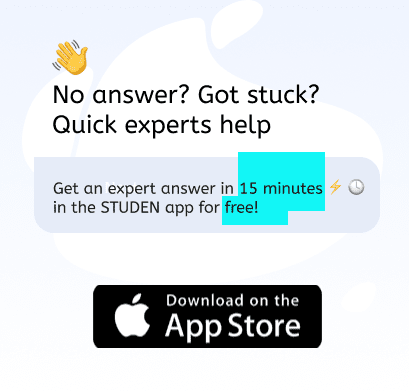
Another question on Computers and Technology

Computers and Technology, 22.06.2019 20:00
Need asap write a short paper describing the history and differences between six sigma, waterfall, agile, and scrum models. understanding these models can give you a good idea of how diverse and interesting it development projects can be. describe what the rationale for them is and describe their key features. describe the history behind their development. at least 400 words
Answers: 1

Computers and Technology, 24.06.2019 13:50
Write a program that performs a simple n-body simulation, called "jumping leprechauns." this simulation involves n leprechauns, numberd 1 to n. it maintains a gold value g_i for each leprechaun i, which begins with each leprechaun starting out with a million dollars worth of gold, that is, g_i = 1000000 for each i = 1,. in addition, the simulation also maintains, for each leprachaun,i, a place on the horizon, which is represented as a double-precision floating point number, x_i. in each iteration of the simulation, the simulation processes the leprachauns in order. processing a leprachaun i during its iteration begins by computing a new place on the horizon for i, which is determined by the assignment:
Answers: 3

Computers and Technology, 25.06.2019 05:00
What should you do if a dialog box covers an area of the screen that you need to see?
Answers: 1

Computers and Technology, 25.06.2019 06:50
Write a program that will askthe user to enter the amount of a purchase. the program should thencompute the state and county sales tax. assume the state sales tax is4 percent and the county sales tax is 2 percent. the program should displaythe amount of the purchase, the state tax, the county sales tax, the total salestax, and the total of the sale. (which is the sum of theamount of purchase plus the total sales tax). hint: use the value 0.02 torepresent 2 percent, and 0.04 to represent 4 percent.
Answers: 2
You know the right answer?
The C library function memset(p, c,n) writes (uint8_t)(c) repeatedly n times begininig at the addres...
Questions

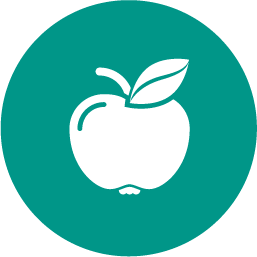
Physics, 06.05.2020 08:21

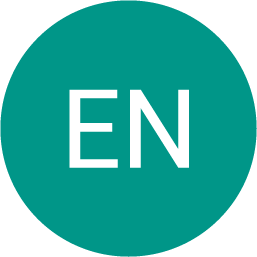

Mathematics, 06.05.2020 08:21


Mathematics, 06.05.2020 08:21

Mathematics, 06.05.2020 08:21

Mathematics, 06.05.2020 08:21

Mathematics, 06.05.2020 08:21

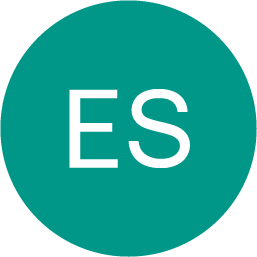

Mathematics, 06.05.2020 08:21

Chemistry, 06.05.2020 08:21



Mathematics, 06.05.2020 08:21
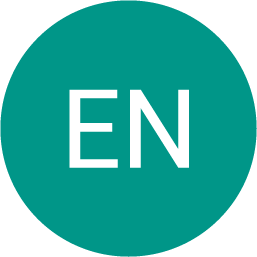
English, 06.05.2020 08:21
