
Computers and Technology, 05.11.2021 09:40 davidoj13
For this exam review lab exercise, you will develop a program that fixes faulty data in a one-dimensional array of integers. Faulty data is represented by a value of 9999, and should be replaced by the average of the two neighboring elements, where integer casting should be applied to non-integer averages (to keep the data stored as integers). If the first or last element is faulty, it should be replaced by the value of its only neighboring element. If two adjacent neighboring elements are faulty anywhere in the array, a message should be printed to screen stating that the data cannot be fixed. The programming tasks have been segmented into levels. It is strongly recommended that you complete all lower level tasks before moving on to a higher level.
A code template has been provided for you that reads in data from the user and prints out the inputted data to screen. DO NOT MODIFY THE TEMPLATE CODE, INSTEAD ONLY ADD FUNCTIONALITY FOR THE LEVEL 1-3 TASKS AS DESCRIBED BELOW.
Programming Tasks
Level 0 Tasks
Simply ensure you submit a code that compiles and runs without any errors and that the functionality of the code template has not been modified. Beyond that, you don't need to do anything else to earn these points. (To be clear, submit the template right now, and you should get at least 50 points.)
Level 1 Tasks
Write the checkData() function, which takes in as parameters an integer data array, the array size, and another integer badValue. The function should return true if there are no elements in the array that contain badValue. The function should return false if the array stores badValue at any element in the array.
Call checkData() from main() to check for faulty data in the array temps. Depending on the result, print to screen either
No Faulty Data!
OR
Fixing Faulty Data...
Write the fixData() function, which has the same parameters as checkData(). The function should fix the data by replacing all elements that are badValue with the average of the two neighboring elements. For Level 1, you can assume that the first and last elements do not contain badValue and that no two consecutive elements contain badValue. The function should return the number of elements that originally stored badValue but have now been fixed.
Call fixData() from main() to fix the data in temps and print the updated array to screen using the provided printData() function.
Level 2 Tasks
Update fixData() for cases where badValue is stored in the first or last element. In these cases, replace the the faulty data with the only neighboring element. For Level 2, you can still assume that no two consecutive elements contain badValue.
Level 3 Tasks
Update fixData() for cases where badValue is stored in two consecutive elements. In these cases, return -1 to main(), where you the following message should be printed to screen (and then the program immediately terminates):
Adjacent elements are faulty. Data cannot be fixed!
For this final (Level 3+) task, print an asterisk (i. e. * ) underneath the elements of the fixed array (printed to screen in the last Level 1 Task) for all elements that originally were faulty, but have now been updated using the neighboring elements. You need to be careful with formatting here: the asterisk should go directly under the highest digit of the number.
Sample Output
Here are some sample outputs for your reference:
A code that achieves Level 1 Tasks:
Input:
12
2
9999
17
9999
-2
22
9999
4
11
9999
8
6
Output:
Enter size of data:
Enter data:
Inputted data:
2 9999 17 9999 -2 22 9999 4 11 9999 8 6
Fixing Faulty Data...
Fixed Data:
2 9 17 7 -2 22 13 4 11 9 8 6
A code that achieves Level 2 Tasks:
Input:
11
9999
17
9999
-2
22
9999
4
11
9999
8
6
Output:
Enter size of data:
Enter data:
Inputted data:
9999 17 9999 -2 22 9999 4 11 9999 8 6
Fixing Faulty Data...
Fixed Data:
17 17 7 -2 22 13 4 11 9 8 6
A code that achieves Level 3 Tasks:
Input:
10
9999
9999
-2
22
9999
4
11
9999
8
6
Output:
Enter size of data:
Enter data:
Inputted data:
9999 9999 -2 22 9999 4 11 9999 8 6
Fixing Faulty Data...
Adjacent elements are faulty. Data cannot be fixed!
A code that achieves Level 3+ Tasks:
Input:
10
9999
-2
22
9999
4
11
8
9999
7
9999
Output:
Enter size of data:
Enter data:
Inputted data:
9999 -2 22 9999 4 11 8 9999 7 9999
Fixing Faulty Data...
Fixed Data:
-2 -2 22 13 4 11 8 7 7 7
* * * *

Answers: 3
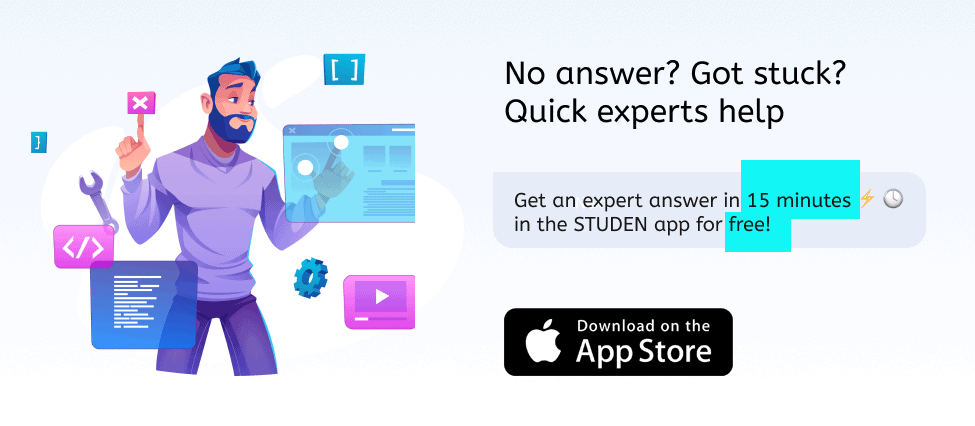
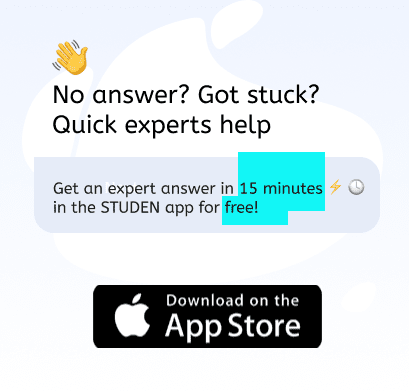
Another question on Computers and Technology

Computers and Technology, 22.06.2019 12:50
You have just been hired as an information security engineer for a large, multi-international corporation. unfortunately, your company has suffered multiple security breaches that have threatened customers' trust in the fact that their confidential data and financial assets are private and secured. credit-card information was compromised by an attack that infiltrated the network through a vulnerable wireless connection within the organization. the other breach was an inside job where personal data was stolen because of weak access-control policies within the organization that allowed an unauthorized individual access to valuable data. your job is to develop a risk-management policy that addresses the two security breaches and how to mitigate these risks.requirementswrite a brief description of the case study. it requires two to three pages, based upon the apa style of writing. use transition words; a thesis statement; an introduction, body, and conclusion; and a reference page with at least two references. use a double-spaced, arial font, size 12.
Answers: 1

Computers and Technology, 23.06.2019 15:00
Idon’t understand the double8 coding problem. it is java
Answers: 1

Computers and Technology, 24.06.2019 07:00
Selective is defined as paying attention to messages that are consistent with one’s attitudes and beliefs and ignoring messages that are inconsistent.
Answers: 1

Computers and Technology, 24.06.2019 13:30
Type the correct answer in the box. spell all words correctly. what is the default margin width on all four sides of a document? by default, the document has a margin on all four sides.
Answers: 1
You know the right answer?
For this exam review lab exercise, you will develop a program that fixes faulty data in a one-dimens...
Questions
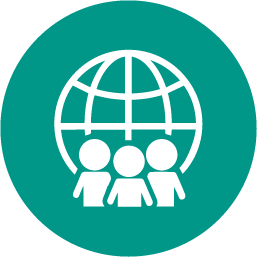

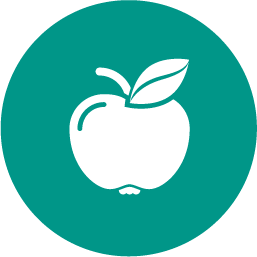
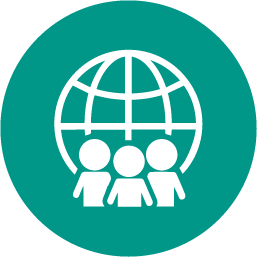
History, 24.07.2019 04:10
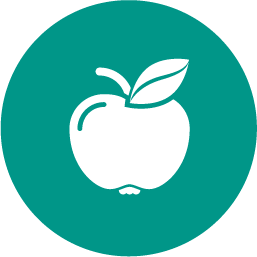
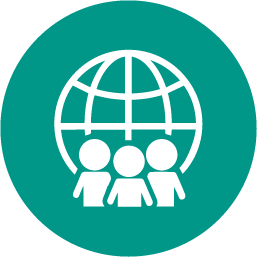
History, 24.07.2019 04:10

Mathematics, 24.07.2019 04:10
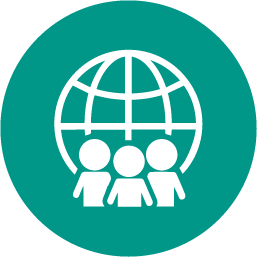
History, 24.07.2019 04:10


Mathematics, 24.07.2019 04:10



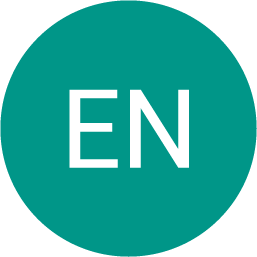

Chemistry, 24.07.2019 04:10
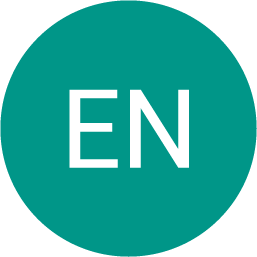

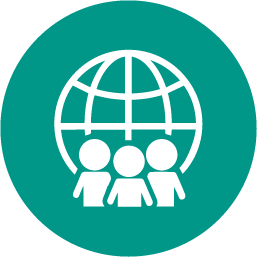
