
Computers and Technology, 24.11.2021 20:00 abelinoperez652
# program-01-steps
Using steps. py in this repository for a starter file, write a Python program to calculate average number of steps per month for a year.
The input file (which you will read from the command line, see the sample program on how to read command line arguments in this repository) contains the number of steps (integer) a person took each day for 1 year, starting January 1. Each line of input contains a single number.
Assume this is NOT a leap year.
Your output must follow this format:
```
The average steps taken in MONTH NAME was 9999.9
```
Where 9999.9 represents that month's average. Be sure to output only a single decimal digit (digit to the right of the decimal)
Be sure to spell out the name of the month. First letter must be caps. For example: June
For a grade of A your program should handle a leap year by looking at the number of steps in the file, if 365 then non leap year, if 366 then leap year.
***commandlines. py***
# Python program to demonstrate
# command line arguments
# notice that the first command line argument that is passed to a program comes after the name of the program file
# it has an array index of 1 in the sys. argv array
# sys. arg[0] is always the name of the python program file
# all command line arguments are strings, so if you want to perform math you have to convert
import sys
# total arguments
n = len(sys. argv)
print("Total arguments passed: %i" % n)
# Arguments passed
print("\nName of Python script: %s" % sys. argv[0])
print("\nArguments passed:")
for i in range(1, n):
print("%s" % sys. argv[i])
# Addition of numbers
Sum = 0
for i in range(1, n):
Sum += int(sys. argv[i])
print("\n\nResult: %i" % Sum)
***steps. py***
# Named constants - create one for each month to store number of days in that month; assume this is NOT a leap year
def main():
# Open the steps file using the first command line argument to get the input file name. For example: python steps. py steps. txt would open the file steps. txt to read the steps
# Display the average steps for each month using a function to calculate and display
# Close the file.
def average_steps(steps_file, month_name, days):
# compute the average number of steps for the given month
# output the results
main()

Answers: 1
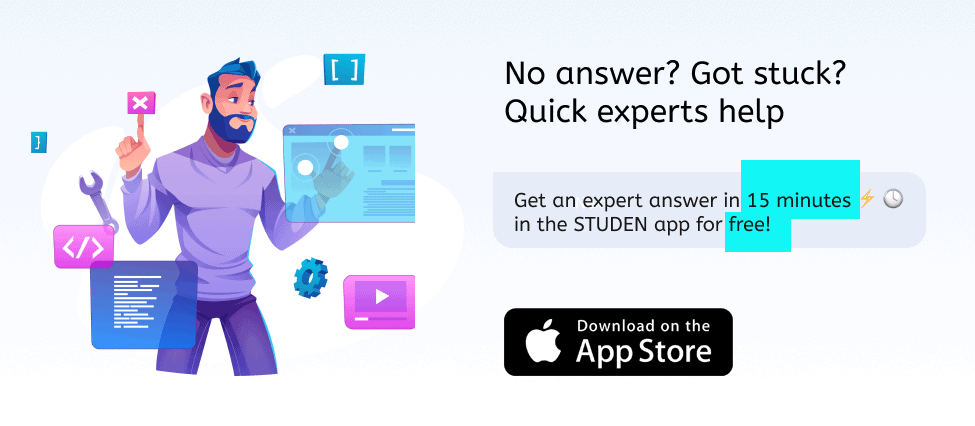
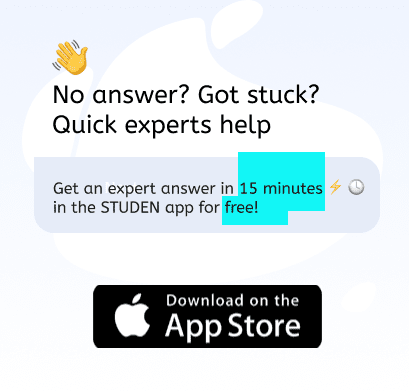
Another question on Computers and Technology

Computers and Technology, 21.06.2019 22:40
State the parts of a variable declaration?
Answers: 2

Computers and Technology, 23.06.2019 05:00
Acompany is inviting design for its new corporate logo from its users. this is an example of ? a. crowdfunding b. crowdvoting c. crowdsourced design d. crowdtracking
Answers: 3

Computers and Technology, 23.06.2019 23:30
The keyboard usually has six rows of keys. which of the following is not one of the key group categories? letter keys number keys control keys graphic keys
Answers: 1

Computers and Technology, 23.06.2019 23:30
What are "open-loop" and "closed-loop" systems
Answers: 1
You know the right answer?
# program-01-steps
Using steps. py in this repository for a starter file, write a Python program t...
Questions


Mathematics, 17.03.2020 18:19

Mathematics, 17.03.2020 18:19


Mathematics, 17.03.2020 18:19

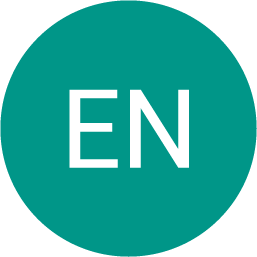

Health, 17.03.2020 18:19
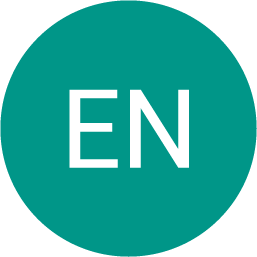
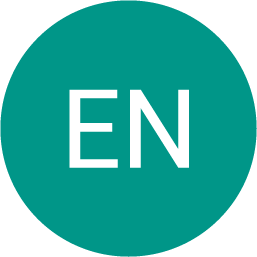
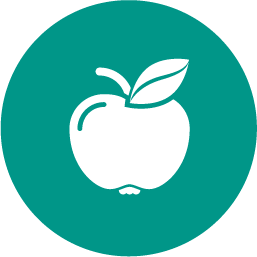
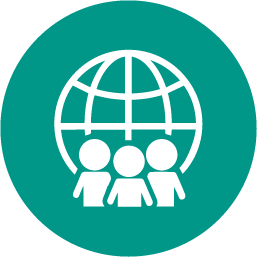
History, 17.03.2020 18:20

Mathematics, 17.03.2020 18:20
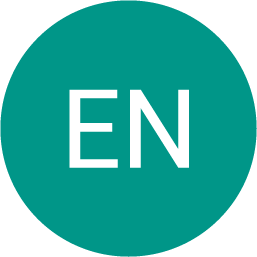
English, 17.03.2020 18:20


Mathematics, 17.03.2020 18:21


Arts, 17.03.2020 18:21

Mathematics, 17.03.2020 18:21
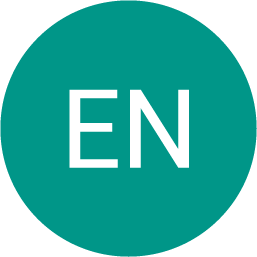
English, 17.03.2020 18:21