
Computers and Technology, 10.12.2021 01:00 zanaplen27
In C++ please.
A company has sales reps that sell two different product lines. The sales amounts for each product are recorded. A commission is paid based on the total sales. The commission is determined as follows:
Total sales Commission
less than $4000 5% of sales
$4000 to less than $8000 7% of sales
$8000 or more 10% of sales
Data for sales reps is stored in a file salesdata. txt. Each line of the file is in this format:
Salesrep name#sales1 sales2
Create a struct to represent a SalesRep with
-Name
-Product1 sales
-Product 2 sales
-Total sales
-Commission
Create an array of type SalesRep in main that can hold up to 20 reps.
Your program will prompt for the name of the file and validate that it exists. Read the data from the file and populate the array. You will need to count the actual number of sales reps in the file. Make sure your code tests that the array is not overfilled.
Create and generate two reports to an output file called reports. txt:
Sales report Print the name, total sales, and commission for all reps entered into the system in the format shown below on sample output. Also calculate and print the total sales and commission of all reps.
Diamond Club Print the name and total sales of all reps with $10000 or more in total sales
Run your program with this file
James T. Kirk#8500 2650
Bruce Wayne#4880 3120
Harry Potter#1500 850
Peter Parker#9800 5100
Clark Kent#2750 1250
Lois Lane#8250 5200
Bob Blue#5125 7500
Steve Green#1200 3000
Jill White#1500 2500
Sam Black#5200 2800
For FULL credit you should create functions to:
-Read the file
-Calculate the commission for an individual
-Generate the sales report
-Generate the diamond club report
It is better to have working functionality WITHOUT functions than to run out of time β¦ (you will lose 10% of the grade).
If you cannot use structures correctly, this program can be done by reading the file twice to generate each report. You will lose 10% of the grade for this option.
For full credit:
you MUST include comments that document the problem solving process
your code must be properly indented
your code will have functions
output should be formatted so that numbers align as in sample output
Make sure the answers are correct! I am purposely not showing the answers
SAMPLE RUN
Enter name of file: s. txt
s. txt not found, re-enter: sales. txt
Reading file
X records read
Report generated
Programmed by yournme
(in reports. txt)
Sales Report
Employee Total Sales Commission
Captain Kirk $ .xx $ .xx
Bruce Wayne $ .xx $ .xx
Harry Potter $ .xx $ .xx
Peter Parker $ .xx $ .xx
Clark Kent $ .xx $ .xx
Total $ .xx $ .xx
Diamond Club Qualified
Name Total Sales
rep $ .xx
This is what I have so far
// Jimmy Nguyen
#include
#include
#include
#include
#include
using namespace std;
struct SalesRep{
string name;
double sales1;
double sales2;
};
typedef struct SalesRep salesrep;
void read_file(string filename , salesrep sales[] , int max_sales){
int current_sales = 0;
ifstream file(filename);
if(file. is_open()){
string line;
while(getline(file , line) && current_sales < max_sales){
string word;
vector first_split;
stringstream ss(line);
while(getline(ss, word , '#')){
first_split. push_back(word);
}
string sale;
vector second_split;
stringstream ss1(first_split[1]);
while(getline(ss1 , sale, ' ')){
second_split. push_back(sale);
}
sales[current_sales].name = first_split[0];
sales[current_sales].sales1 = stod(second_split[0]);
sales[current_sales].sales2 = stod(second_split[1]);
current_sales += 1;
}
}
file. close();
}
void display(salesrep sales[] , int max_sales){
double overall_sales = 0;
double overall_comm = 0;
cout<<"Employee\t\tTotal Sales\t\t Commission\n";
for(int i=0;i
double total_sales = sales[i].sales1 + sales[i].sales2;
overall_sales += total_sales;
double commission;
overall_comm += commission;
if(total_sales < 4000){
commission = total_sales * 0.05;
}
else if(total_sales < 8000){
commission = total_sales * 0.07;
}
else{
commission = total_sales * 0.1;
}
cout<
}
cout<<"\nTotal\t\t\t$"<
}
int main(){
string filename = "salesdata. txt";
int max_sales = 20;
salesrep sales[max_sales];
read_file(filename , sales , max_sales);
display(sales , max_sales);
return 0;
}

Answers: 2
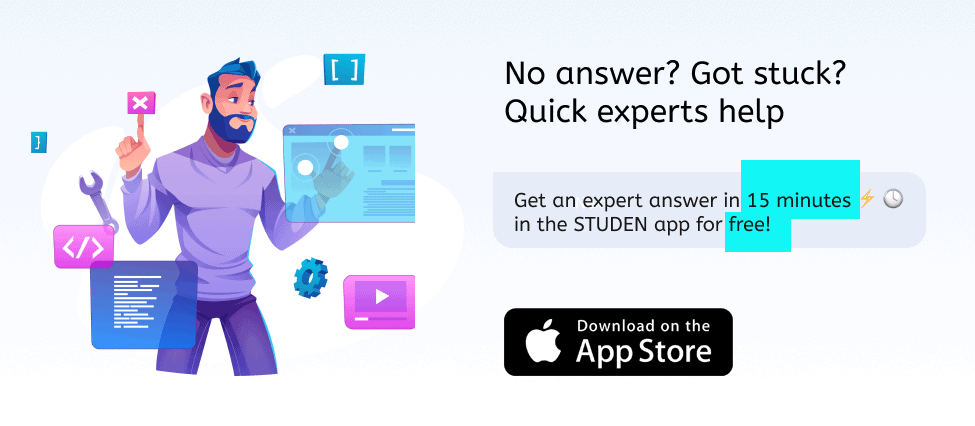
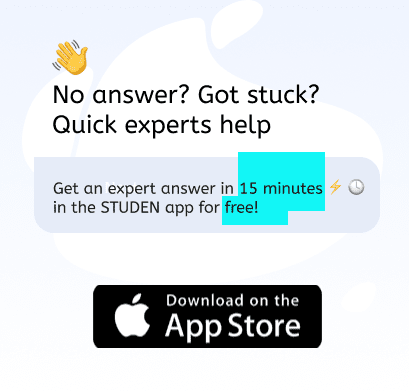
Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:30
Which of the following commands is more recommended while creating a bot?
Answers: 1

Computers and Technology, 23.06.2019 04:31
Cloud computing service providers manage different computing resources based on the services they offer. which resources do iaas and paas providers not manage? iaas providers do not manage the for the client, whereas paas providers usually do not manage the for their clients. iaas- storage server operating system network paas- applications interafce storage vertualiation
Answers: 2

Computers and Technology, 24.06.2019 12:40
Match the feature to the network architecture. expensive to set up useful for a small organization easy to track files has a central server inexpensive to set up difficult to track files useful for a large organization does not have a central server client- server network peer-to-peer network
Answers: 3

Computers and Technology, 24.06.2019 13:00
What are some websites that you can read manga (ex: manga rock)
Answers: 1
You know the right answer?
In C++ please.
A company has sales reps that sell two different product lines. The sales amounts f...
Questions


Geography, 14.10.2019 13:50
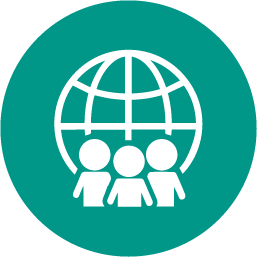
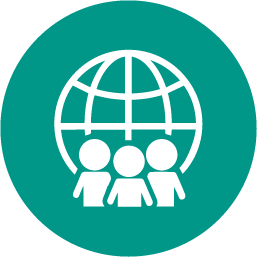

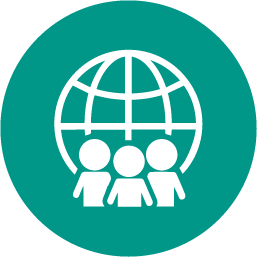
History, 14.10.2019 13:50

Biology, 14.10.2019 13:50

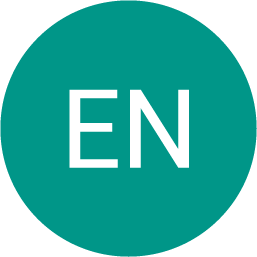

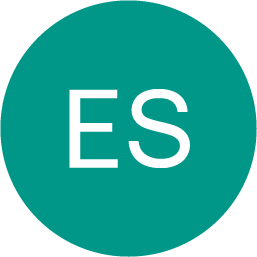
Spanish, 14.10.2019 13:50
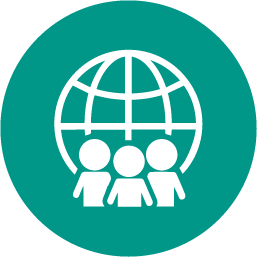

Geography, 14.10.2019 13:50
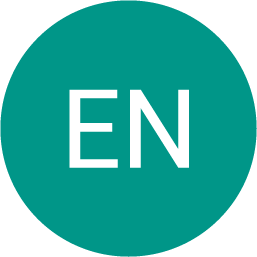

Computers and Technology, 14.10.2019 13:50
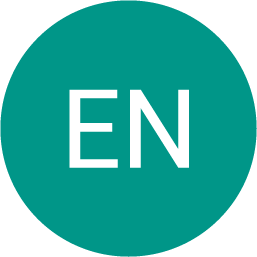
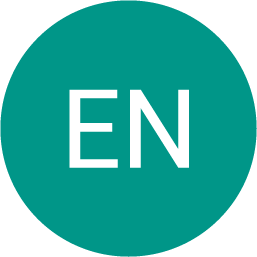
English, 14.10.2019 13:50

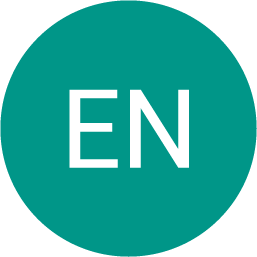
English, 14.10.2019 13:50

Mathematics, 14.10.2019 13:50