
Computers and Technology, 15.12.2021 19:40 icecreamisgood4u
In java
write the code in order to find the breadth first search of the graph from the adjacency matrix.
this question has been asked and all of the tutors are copy and pasting the incorrect answer please do not do that
the first cmd line arg is a text file is called g0.txt and it should have this in it
8
0 1
3 0
3 4
3 5
1 4
2 1
7 4
4 6
5 6
7 6
2 7
the 2nd cmd line arg is 4
and the 3rd cmd line arg is 2
with these the final output should be
8
0 1
3 0
3 4
3 5
1 4
2 1
7 4
4 6
5 6
7 6
2 7
4, 6, 7, 1, 3, 5, 2, 0
2, 7, 1, 4, 6, 0, 3, 5
import java. io.*;
import java. util.*;
public class UndirectedGraph {
private class Vertex {
private EdgeNode edges1;
private EdgeNode edges2;
private boolean queued;
private Vertex() {
edges1 = null;
edges2 = null;
queued=false;
}
private Vertex(EdgeNode e1, EdgeNode e2) {
edges1 = e1;
edges2 = e2;
}
}
private class EdgeNode {
private int vertex1;
private int vertex2;
private EdgeNode next1;
private EdgeNode next2;
private EdgeNode(int v1, int v2) {
//PRE: v1 < v2
vertex1 = v1;
vertex2 = v2;
}
}
private Vertex[] g;
public UndirectedGraph(int size) {
g = new Vertex[size];
for (int i = 0; i < size; i++) {
g[i] = new Vertex();
}
}
public void addEdge(int v1, int v2) {
//if the vertices aren't in accending order, swap
if(v1 > v2) {
int temp = v1;
v1 = v2;
v2 = temp;
}
//if a vertex is null, instantiate it
if(g[v1] == null) {
g[v1] = new Vertex(new EdgeNode(v1, v2), null);
}
//if edges1 is still null, instantiate
else if(g[v1].edges1 == null) {
g[v1].edges1 = new EdgeNode(v1, v2);
}
//else add edge to the list in order
else {
EdgeNode temp = g[v1].edges1;
//traverse list until you come to the end, or you come to the appropriate location
while(temp. next1 != null && temp. vertex2 < v2) {
temp = temp. next1;
}
EdgeNode e = new EdgeNode(v1, v2);
//add before temp if v2 is less than vertex2
if(temp. vertex2 > v2) {
e. next1 = temp;
if(g[v1].edges1 != null && g[v1].edges1.vertex2 < v2) {
EdgeNode temp2 = g[v1].edges1;
//goto end of existing list, but before place of insertion
while(temp2.next1 != null && temp2.next1 != temp)
temp2 = temp2.next1;
temp2.next1 = e;
}
else
g[v1].edges1 = e;
}
//else add after temp
else {
if (temp. next1 != null) {
e. next1 = temp. next1;
}
temp. next1 = e;
}
}
//if a vertex is null, instantiate it
if(g[v2] == null) {
g[v2] = new Vertex(null, new EdgeNode(v1, v2));
}
//if edges2 is still null, instantiate
else if(g[v2].edges2 == null) {
g[v2].edges2 = new EdgeNode(v1, v2);
}
//else add edge to the list in order
else {
EdgeNode temp = g[v2].edges2;
//traverse list until you come to the end, or you come to the appropriate location
while(temp. next2 != null && temp. vertex1 < v1) {
temp = temp. next2;
}
EdgeNode e = new EdgeNode(v1, v2);
//add before temp if v1 is less than vertex1
if(temp. vertex1 > v1) {
e. next2 = temp;
if(g[v2].edges2 != null && g[v2].edges2.vertex1 < v1) {
EdgeNode temp2 = g[v2].edges2;
//goto end of existing list, but before place of insertion
while(temp2.next2 != null && temp2.next2 != temp)
temp2 = temp2.next2;
temp2.next2 = e;
}
else
g[v2].edges2 = e;
}
//else add after temp
else {
if (temp. next2 != null) {
e. next2 = temp. next2;
}
temp. next2 = e;
}
}
}
public void printBFS(int start) {
//Print a comma delimited list of nodes in BFS order
}
public void printGraph() {
for(int i = 0; i < g. length; i++) {
EdgeNode temp1 = g[i].edges1;
EdgeNode temp2 = g[i].edges2;
System. out. printf("%s ", i);
while(temp1 != null) {
System. out. printf("%s ", temp1.vertex2);
temp1 = temp1.next1;
}
System. out. print("\n ");
while(temp2 != null) {
System. out. printf("%s ", temp2.vertex1);
temp2 = temp2.next2;
}
System. out. println('\n');
}
}
public static void main(String args[]) throws IOException {
BufferedReader b = new BufferedReader(new FileReader(args[0]));
String line = b. readLine();
int numNodes = Integer. parseInt(line);
UndirectedGraph g = new UndirectedGraph(numNodes);
line = b. readLine();
while (line != null) {
Scanner scan = new Scanner(line);
g. addEdge(scan. nextInt(), scan. nextInt());
line = b. readLine();
}
g. printGraph();
g. printBFS(Integer. parseInt(args[1]));
g. printBFS(Integer. parseInt(args[2]));
}
}

Answers: 2
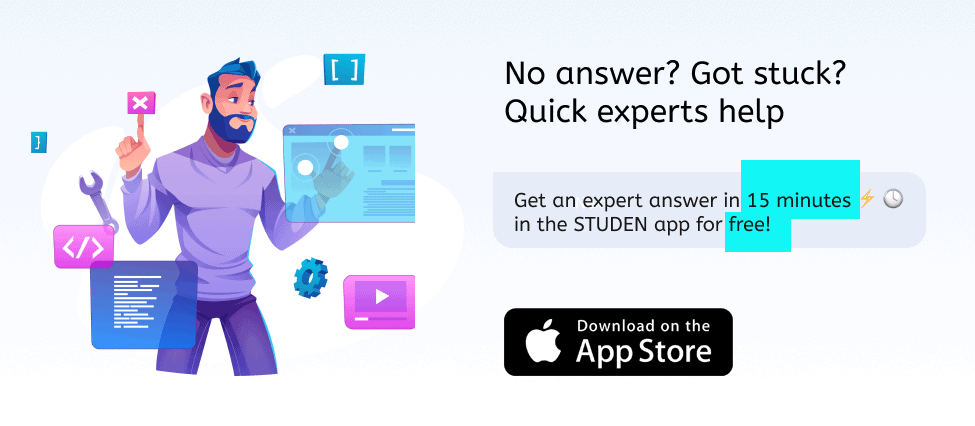
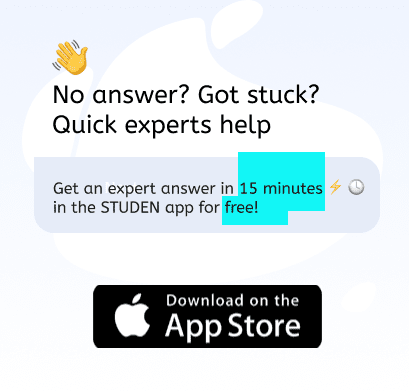
Another question on Computers and Technology

Computers and Technology, 22.06.2019 23:50
List a few alternative options and input and output over the standerd keyboard and monitor. explain their functioning in details.
Answers: 2

Computers and Technology, 23.06.2019 14:00
What is html ? give a small description about html
Answers: 2


Computers and Technology, 23.06.2019 23:30
A. in packet tracer, only the server-pt device can act as a server. desktop or laptop pcs cannot act as a server. based on your studies so far, explain the client-server model.
Answers: 2
You know the right answer?
In java
write the code in order to find the breadth first search of the graph from the adjacency m...
Questions

Mathematics, 02.06.2021 20:00

Chemistry, 02.06.2021 20:00


Biology, 02.06.2021 20:00
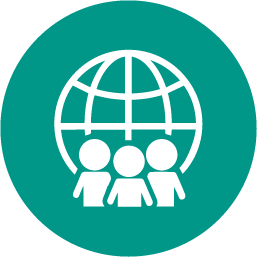
History, 02.06.2021 20:00

Mathematics, 02.06.2021 20:00

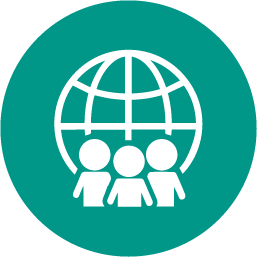
History, 02.06.2021 20:00
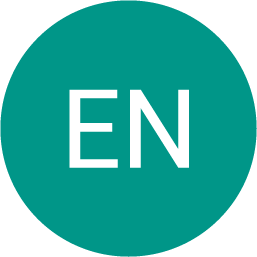

Mathematics, 02.06.2021 20:00
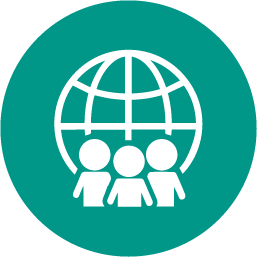

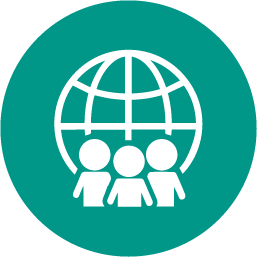
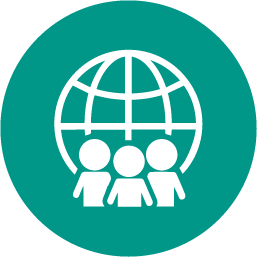
History, 02.06.2021 20:00

Mathematics, 02.06.2021 20:00


Mathematics, 02.06.2021 20:00

Arts, 02.06.2021 20:00
