
Computers and Technology, 16.12.2021 21:30 katelynzaro
Create a HighestGrade application that prompts the user for five grades between 0 and 100 points and stores
the grades in an ArrayList. HighestGrade then traverses the grades to determine the highest grade and then
displays the grade along with an appropriate message.
For HighestGrade, you need to use an ArrayList. You do not need to declare any Integer elements because you do not need to use a .get since you don’t need to find the index position of the grades. Use two loops. The first loop will ask the user to enter a grade. The second loop will determine the highest grade
import java. util. ArrayList;
public class DataArrayList {
public static void main(String[] args) {
ArrayList numbers = new ArrayList ();
Integer element, element1, element2;
int sum = 0;
numbers. add(new Integer(5));
numbers. add(new Integer(2));
/* compare values */
element1 = numbers. get(0);
element2 = numbers. get(1);
if (element1.compareTo(element2) == 0) {
System. out. println("The elements have the same value.");
} else if (element1.compareTo(element2) < 0) {
System. out. println("element1 value is less than element2.");
} else {
System. out. println("element1 value is greater than element2.");
}
/* sum values */
for (Integer num : numbers) {
element = num;
sum += element. intValue(); //use int value for sum
}
System. out. println("Sum of the elements is: " + sum);
}
}

Answers: 3
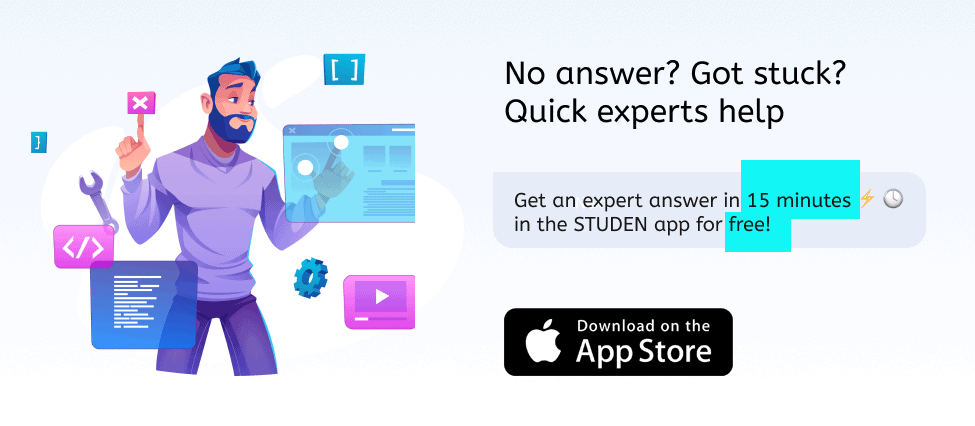
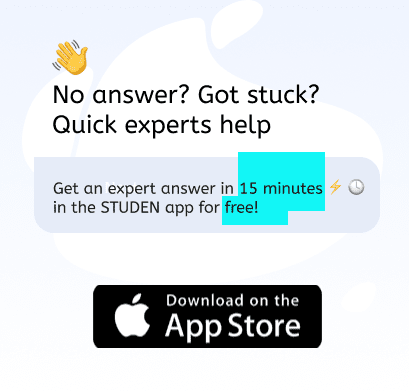
Another question on Computers and Technology

Computers and Technology, 22.06.2019 22:40
Least square fit to polynomial write a function leastsquarefit3pol that solves a linear system of equations to find a least squares fit of a third order polynomial to an experimental data set given as two row arrays. the function leastsquarefit3pol must explicitly solve a set of linear equations and cannot use polyfit. there should be no restriction on the size of the problem that can be solved.
Answers: 1


Computers and Technology, 23.06.2019 05:20
Which operating system is a version of linux?
Answers: 1

Computers and Technology, 23.06.2019 06:30
How do you write an argumentative essay about the importance of free enterprise ?
Answers: 1
You know the right answer?
Create a HighestGrade application that prompts the user for five grades between 0 and 100 points and...
Questions

Mathematics, 26.01.2020 13:31
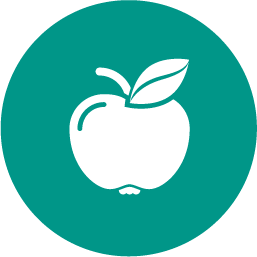
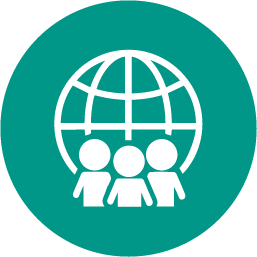
Social Studies, 26.01.2020 13:31



Mathematics, 26.01.2020 13:31
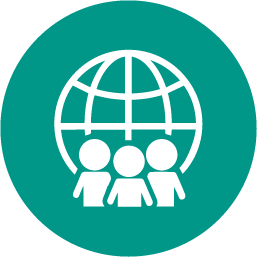
History, 26.01.2020 13:31

Business, 26.01.2020 13:31


Biology, 26.01.2020 13:31
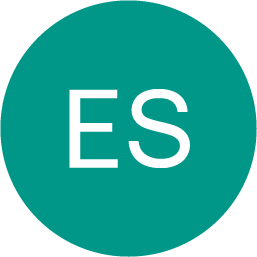
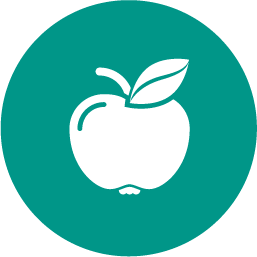
Physics, 26.01.2020 13:31
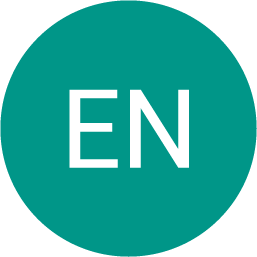

Mathematics, 26.01.2020 13:31

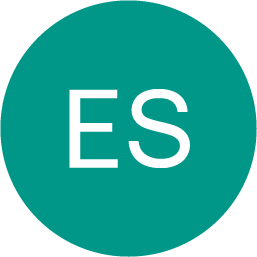
Spanish, 26.01.2020 13:31


Mathematics, 26.01.2020 13:31

Mathematics, 26.01.2020 13:31

Mathematics, 26.01.2020 13:31