
Computers and Technology, 27.01.2022 20:30 amcd2002
Write a program that will automatically generate and solve mazes.
Each time the program is run, it will generate and print a new random maze and solution. You will use the disjoint set (union-find) data structure, depth-first search (DFS), and breadth-first search (BFS).
Generating a Maze:
Conceptually, to generate a maze, first start with a grid of rooms with walls between them. The grid contains r rows and c columns for a total of r*c rooms. For example, Figure 1 is a 4x4 grid of 16 rooms. The missing walls at the top left and bottom right of the maze border indicate the start and finish rooms of the maze.
Now, begin removing interior walls to connect adjacent rooms. The difficultly in generating a good maze is in choosing which walls to remove. Walls should be removed to achieve the following maze characteristics:
1. Randomized: To generate unpredictable different mazes, walls should be selected randomly as candidates for removal. For randomization, you must use the random generator function to generate random numbers.
2. Single solution: There should be only one path between the start room and the finish room.
Unnecessarily removing too many walls will make the maze too easy to solve. Therefore, a wall should not be removed if the two rooms on either side of the wall are already connected by some other path. For example, in Figure 2, the wall between a and f should not be removed because walls have previously been removed that create a path between a and f through b, c, d, e. Use the disjoint set data structure to determine room connected-ness.
3. Fully connected: Enough walls must be removed so that every room is reachable from the start room. There must be no rooms or areas that are completely blocked off from the rest of the maze. Figure 3 shows an example generated maze.
Solving the Maze:
After generating a maze, your program should then solve the maze first using DFS and then again using BFS. Each search algorithm will begin at the start room and search for the finish room by traversing wall openings. The search should terminate as soon as the finish room is found. For each search algorithm, you will output the order in which rooms where visited and indicate the shortest solution path from start to finish.
Design:
You will likely represent the maze as a graph data structure, where rooms are nodes and removed walls are edges between nodes. Since the size of the graph is known at startup, a 2 dimensional array-based implementation that mimics the grid structure may work well. To randomly select walls for removal, you will also need to maintain a separate list of walls eligible for removal. As randomly selected walls are removed from the maze or determined to be ineligible for removal (because of rule 2 above), they are eliminated from the wall list. This places a tight upper bound on the number of iterations of the wall-removal loop.
You must use the disjoint set data structure for the union and find operations on rooms when generating the maze. It is required that you implement the weighted union rule and the path compression technique for maximum efficiency. Rooms that are connected by some path are in the same set. The find operation reveals whether two rooms are already connected by some path. When removing a wall, the union operation is used to join the two sets together. The maze generator is done when there is only one set left, indicating that all rooms are connected. The disjoint set data structure enables efficient processing of the union and find operations, so that maze generation is fast.
Input:
The program should be named βmazeβ and should accept the number of rows r and columns c of the maze as command-line arguments. If no command line arguments are given, it should default to 20 rows by 20 columns. The following invocation would create a maze that is 10 rows by 20 columns:
java maze 10 20
Output:
The program should print to standard-out the maze, then the DFS solution, then the BFS solution. The maze is printed in ASCII using the vertical bar β|β and dash β-β characters to represent walls, β+β for corners, and space character for rooms and removed walls. The start and finish rooms should have exterior openings as shown.
For the DFS and BFS solutions, the maze should be output twice for each algorithm. The first maze output for each algorithm should show the order that the rooms were visited by the algorithm. The maze should be printed exactly as above except that rooms should be printed with the low-order digit of the visitation order number. The start room is β0β. Unvisited rooms should remain a space character. The second maze output for each algorithm should show the shortest solution path, using hash β#β character for rooms and wall openings on the solution path.

Answers: 1
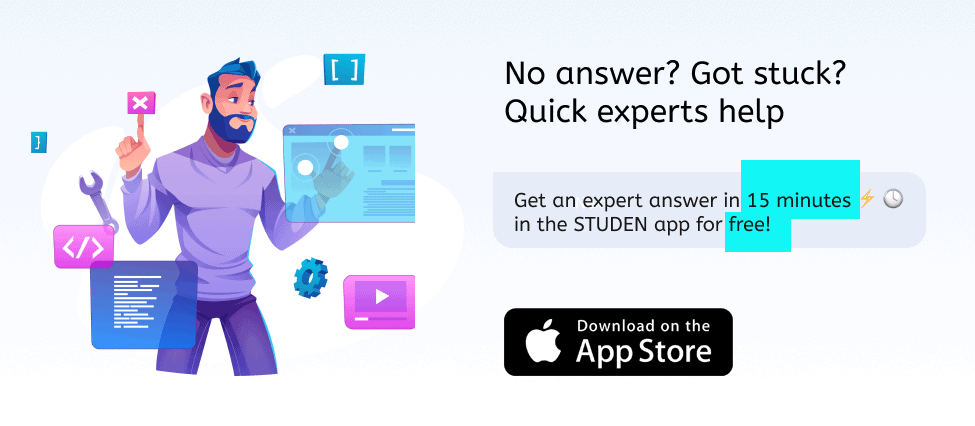
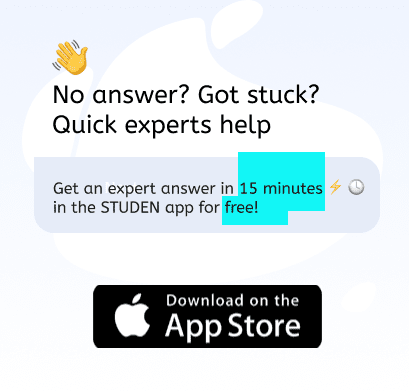
Another question on Computers and Technology

Computers and Technology, 22.06.2019 02:50
Which of the following had the greatest influence on opening the internet to the generly public
Answers: 1

Computers and Technology, 22.06.2019 18:30
What is outfitting a workplace with video in a technology
Answers: 2

Computers and Technology, 23.06.2019 00:00
How do we use the sumif formula (when dealing with different formats) ?
Answers: 1

Computers and Technology, 23.06.2019 01:30
For a typical middle-income family, what is the estimated cost of raising a child to the age of 18? $145,500 $245,340 $304,340 $455,500
Answers: 2
You know the right answer?
Write a program that will automatically generate and solve mazes.
Each time the program is run, it...
Questions


Mathematics, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40

Business, 14.04.2021 22:40
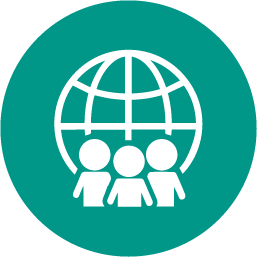
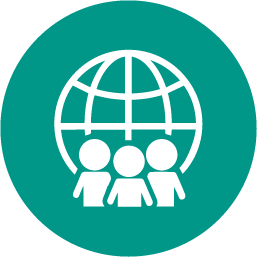

Mathematics, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40


Mathematics, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40

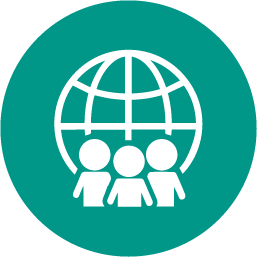
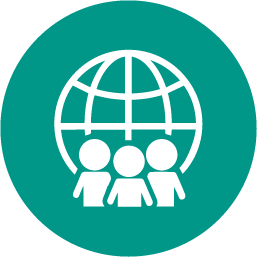
History, 14.04.2021 22:40

Mathematics, 14.04.2021 22:40

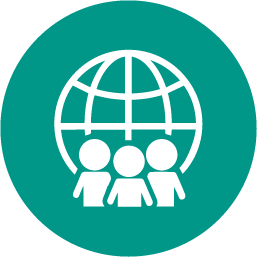

Mathematics, 14.04.2021 22:40