
Computers and Technology, 13.03.2022 18:40 staffordkimberly
I am trying to implement __add__ but I am having trouble what to write next. How can it be implemented? Realted methods are given along with __add__:
from typing import Any
__author__ = 'bailey'
__version__ = '1.00'
__date__ = 'February 21, 2022'
"""
History:
1.00 Feb. 21, 2022 - Initial release.
"""
class Polynomial:
class TermNode:
def __init__(self, coefficient: int, exponent: int) -> None:
"""Initialize this node to represent a polynomial term with the
given coefficient and exponent.
Raise ValueError if the coefficent is 0 or if the exponent
is negative.
"""
if coefficient == 0:
raise ValueError("TermNode: zero coefficient")
if exponent < 0:
raise ValueError("TermNode: negative exponent")
self. coeff = coefficient
self. exp = exponent
self. next = None
def __init__(self, coefficient: int = None, exponent: int = 0) -> None:
"""Initialize this Polynomial with a single term constructed from the
coefficient and exponent.
If one argument is given, the term is a constant coefficient
(the exponent is 0).
If no arguments are given, the Polynomial has no terms.
# Polynomial with no terms:
>>> p = Polynomial()
>>> print(p._head)
None
>>> print(p._tail)
None
# Polynomial with one term (a constant):
>>> p = Polynomial(12)
>>> p._head. coeff
12
>>> p._head. exp
0
>>> print(p._head. next)
None
# Polynomial with one term:
>>> p = Polynomial(12, 2)
>>> p._head. coeff
12
>>> p._head. exp
2
>>> print(p._head. next)
None
"""
# A polynomial is stored as a singly linked list. Each node stores
# one term, with the nodes ordered in descending order, based on the
# exponent. (The head node is the term with the highest exponent,
# and the tail node is the term with the lowest exponent.)
if coefficient is None and exponent == 0:
self._head = None
else:
self._head = Polynomial. TermNode(coefficient, exponent)
self._tail = self._head
def __str__(self) -> str:
"""Return a string representation of this polynomial.
# Polynomial with no terms:
>>> p = Polynomial()
>>> str(p)
''
# Polynomial with one term (a constant):
>>> p = Polynomial(12)
>>> str(p)
'12'
# Polynomials with one term:
>>> p = Polynomial(12, 1)
>>> str(p)
'12x'
>>> p = Polynomial(12, 2)
>>> str(p)
'12x^2'
# See __add__ for string representations of polynomials with
# more than one term.
"""
s = ''
node = self._head
while node is not None:
s += str(node. coeff)
if len(s):
s += '+'
if node. exp == 1:
s += 'x'
elif node. exp > 1:
s += 'x^' + str(node. exp)
node = node. next
return s
def __add__(self, rhs: 'Polynomial') -> 'Polynomial':
""" Return a new Polynomial containing the sum of this polynomial
and rhs.
Raise ValueError if either polynomial has no terms.
>>> p1 = Polynomial(12, 2)
>>> p2 = Polynomial(-3, 1)
>>> p3 = Polynomial(7)
>>> p1 + p2
12x^2-3x
>>> p1 + p3
12x^2+7
>>> p1 + p2 + p3 # Equivalent to (p1 + p2) + p3
12x^2-3x+7
>>> p2 = Polynomial(3, 1)
>>> p1 + p2 + p3
12x^2+3x+7
"""
poly = Polynomial. Termnode()
node = self._head
node2 = rhs._head
while node or node2 is not None:
if node. exp == node2.exp:
coeff = node. coeff + node2.coeff

Answers: 3
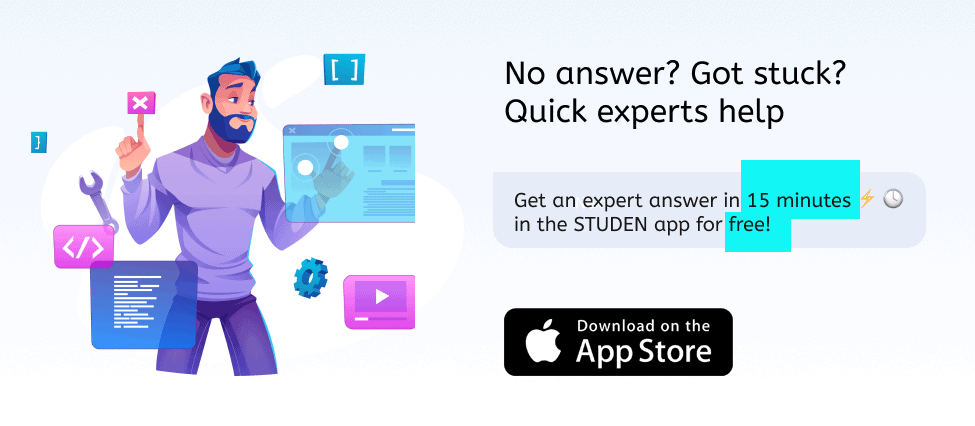
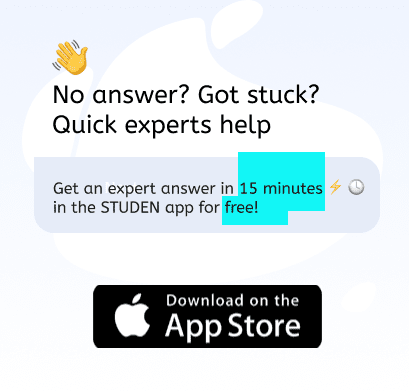
Another question on Computers and Technology

Computers and Technology, 22.06.2019 04:00
When you collaborate or meet with a person or group online, it is called
Answers: 1

Computers and Technology, 22.06.2019 20:00
Need asap write a short paper describing the history and differences between six sigma, waterfall, agile, and scrum models. understanding these models can give you a good idea of how diverse and interesting it development projects can be. describe what the rationale for them is and describe their key features. describe the history behind their development. at least 400 words
Answers: 1

Computers and Technology, 23.06.2019 06:20
What is a point-in-time measurement of system performance?
Answers: 3

Computers and Technology, 23.06.2019 15:20
An ou structure in your domain has one ou per department, and all the computer and user accounts are in their respective ous. you have configured several gpos defining computer and user policies and linked the gpos to the domain. a group of managers in the marketing department need different policies that differ from those of the rest of the marketing department users and computers, but you don't want to change the top-level ou structure. which of the following gpo processing features are you most likely to use? a, block inheritance b, gpo enforcement c, wmi filtering d, loopback processing
Answers: 3
You know the right answer?
I am trying to implement __add__ but I am having trouble what to write next. How can it be implement...
Questions
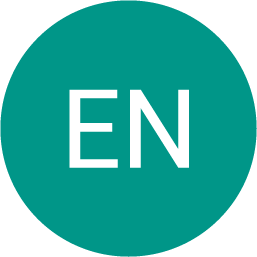
English, 20.09.2019 06:50
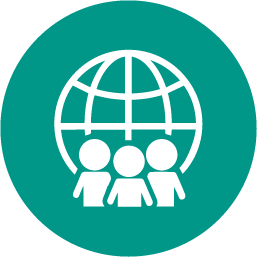
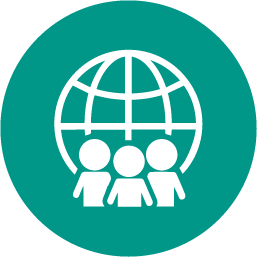

Mathematics, 20.09.2019 06:50
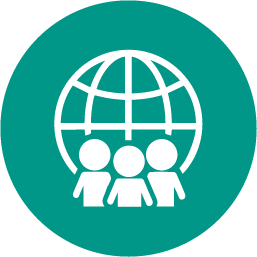
Social Studies, 20.09.2019 06:50
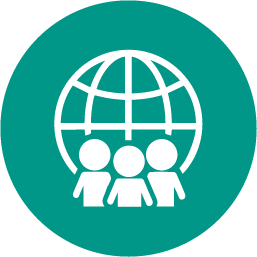
Social Studies, 20.09.2019 06:50
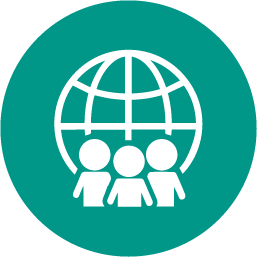
History, 20.09.2019 06:50
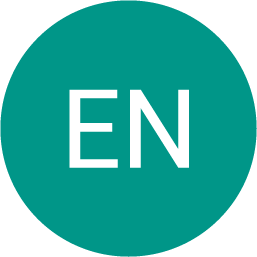

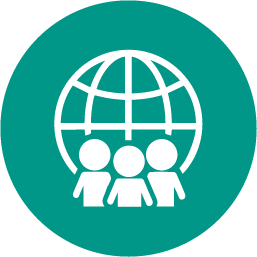
Social Studies, 20.09.2019 06:50

Mathematics, 20.09.2019 06:50

Mathematics, 20.09.2019 06:50


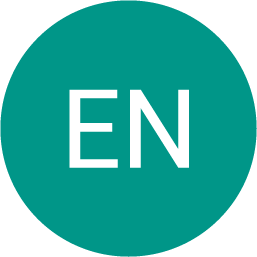


Mathematics, 20.09.2019 06:50

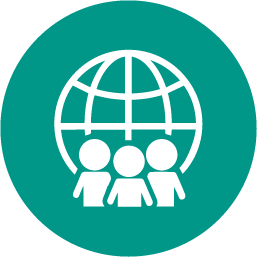
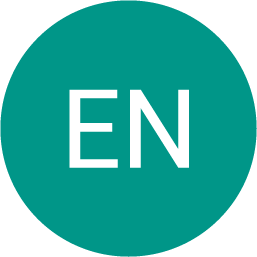
English, 20.09.2019 06:50