
Computers and Technology, 16.04.2020 03:37 needhelpwithHW10
The shell project will compile and execute, and even read a stream file and populate the list with data from a string file.
Steps
You are then asked to complete the following TODO action items:
Review the FileStream Class and:
in the readlist method add the statements to add a string to the array list
in the writeData method add the statement to write the string to the file
Review the PizzaFileIO class and:
In the writeData method add code to add the missing order fields to the record
In the readData method add code to add the missing fields to the order object
Review the OrderList class
In the remove method add statements to remove the order from the list
In the save method add the statement to write the array list to the file.
Graphical User Interface
Update the given graphical user interface to:
Save the list in the Order list to a file using both types of file I/O (streams and objects).
Retrieve the list from the file and display the list
Add a menu items Save Orders and Retrieve Orders
Update retrieveOrders method to retrieve the list from the orderList object.
in OrderList. java;
public void remove(PizzaOrder aOrder) {
if (aOrder != null) {
//TODO: remove the order object from the arraylist
}
}
public int save() throws IOException {
int count = 0;
//TODO: write the array list to file
return count;
}
public int retrieve(DefaultListModel listModel) throws IllegalArgumentException, IOException {
int count = 0;
orderList = streamFile. readData();
if (!orderList. isEmpty()) {
listModel. clear();
count = orderList. size();
for(PizzaOrder order : orderList) {
listModel. addElement(order);
}
}
public void remove(PizzaOrder aOrder) {
if (aOrder != null) {
//TODO: remove the order object from the arraylist
}
}
public int save() throws IOException {
int count = 0;
//TODO: write the array list to file
return count;
}
//Pizza_Main. java
private void retrieveOrders() throws IllegalArgumentException, IOException {
int count = 0;
//TODO: add statement to retrieved the order list (remember to pass in the list model)
String message = count + " pizza orders retreived from file: " + orderList. getFileName();
JOptionPane. showMessageDialog(null, message, "Orders Retrieved", JOptionPane. PLAIN_MESSAGE);
}
//FileStream. java
public static Boolean writeData(String data, String fileName) throws IOException
{
Boolean success;
try
{
FileWriter dataFile = new FileWriter(fileName, false);
try (PrintWriter output = new PrintWriter(dataFile)) {
//TODO: provide the statement to write the entire data string to the file
}
success = true;
}
catch (IOException ioe)
{
JOptionPane. showMessageDialog(null, ioe. getMessage(), "File Write Error", JOptionPane. ERROR);
success = false;
}
return success;
}
public static ArrayList readList(String fileName) throws FileNotFoundException, IllegalArgumentException, IOException
{
ArrayList dataList = new ArrayList<>();
String str;
try {
String file = checkFile(fileName);
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
while ((str = br. readLine()) != null)
{
//TODO: write the statement to add the string to the arrayList
}
}
}
catch (IOException ioe)
{
JOptionPane. showMessageDialog(null, ioe. getMessage(), "File Read Error", JOptionPane. ERROR);
dataList = null;
}
return dataList;
}
//FileStream. java
public static Boolean writeData(String data, String fileName) throws IOException
{
Boolean success;
try
{
FileWriter dataFile = new FileWriter(fileName, false);
try (PrintWriter output = new PrintWriter(dataFile)) {
//TODO: provide the statement to write the entire data string to the file
}
success = true;
}
catch (IOException ioe)
{
JOptionPane. showMessageDialog(null, ioe. getMessage(), "File Write Error", JOptionPane. ERROR);
success = false;
}
return success;
}
try {
String file = checkFile(fileName);
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
while ((str = br. readLine()) != null)
{
//TODO: write the statement to add the string to the arrayList
}
//PizzaFileO. java
if(!orderList. isEmpty()) {
for(PizzaOrder aOrder:orderList) {
count++;
recordList. append(aOrder. getFirstName());
recordList. append(DELIMTER);
recordList. append(aOrder. getLastName());
recordList. append(DELIMTER);
recordList. append(aOrder. getPizzaSize());
recordList. append(DELIMTER);
recordList. append(aOrder. getCheese());
recordList. append(DELIMTER);
//TODO, write the code to: (remember to separate the fields by the delimiter)
//1. add the statement to add the sausage value to the record
//2. add the statement to add the ham value to the record
//3. add the statements to add the total value to the record
aOrder. setCheese(Boolean. valueOf(row. nextToken()));
//TODO: add statements to:
//1. add the sausage value to the order object
//2. add the ham order to the object
//3. add the total value to the order (keeping in mind you have to "parse" the value into a double
//4. add the order to the array list
}
}
}

Answers: 1
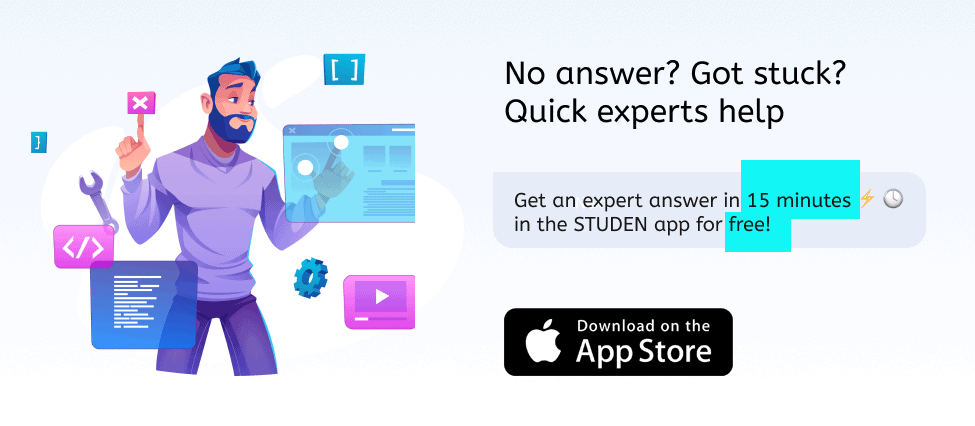
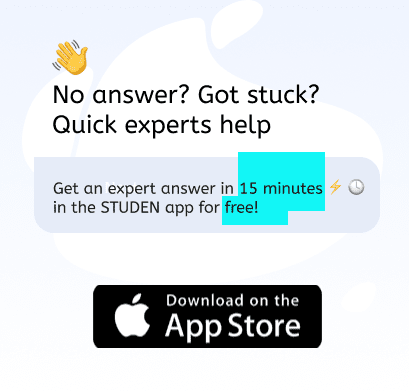
Another question on Computers and Technology

Computers and Technology, 22.06.2019 03:10
Write a program that begins by reading in a series of positive integers on a single line of input and then computes and prints the product of those integers. integers are accepted and multiplied until the user enters an integer less than 1. this final number is not part of the product. then, the program prints the product. if the first entered number is negative or 0, the program must print “bad input.” and terminate immediately. next, the program determines and prints the prime factorization of the product, listing the factors in increasing order. if a prime number is not a factor of the product, then it must not appear in the factorization. sample runs are given below. note that if the power of a prime is 1, then that 1 must appear in t
Answers: 3

Computers and Technology, 22.06.2019 13:50
The instruction ishl (shift left integer) exists in jvm but not in ijvm. it uses the top two values on the stack, replacing the two with a single value, the result. the sec- ond-from-top word of the stack is the operand to be shifted. its content is shifted left by a value between 0 and 31, inclusive, depending on the value of the 5 least signifi- cant bits of the top word on the stack (the other 27 bits of the top word are ignored). zeros are shifted in from the right for as many bits as the shift count. the opcode for ishl is 120 (0x78).a. what is the arithmetic operation equivalent to shifting left with a count of 2? b. extend the microcode to include this instruction as a part of ijv.
Answers: 1

Computers and Technology, 23.06.2019 00:30
Quick pl which one of the following is considered a peripheral? a software b mouse c usb connector d motherboard
Answers: 1

Computers and Technology, 23.06.2019 16:30
Monica and her team have implemented is successfully in an organization. what factor leads to successful is implementation? good between different departments in an organization leads to successful is implementation.
Answers: 1
You know the right answer?
The shell project will compile and execute, and even read a stream file and populate the list with d...
Questions





Mathematics, 04.07.2020 18:01

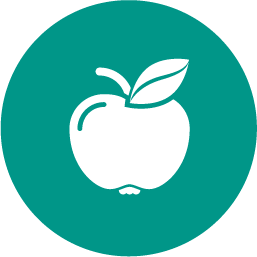






Mathematics, 04.07.2020 18:01
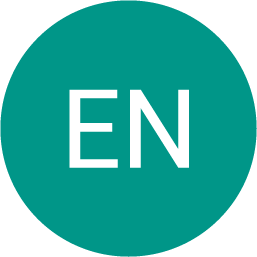


Computers and Technology, 04.07.2020 18:01


Mathematics, 04.07.2020 18:01

Computers and Technology, 04.07.2020 18:01