
Computers and Technology, 18.08.2020 17:01 NeverEndingCycle
The Distance class will represent a distance in some number of feet and inches. This Distance object must always be positive and never contain an inch value greater than or equal to 12 (12 inches equals 1 foot). It is your job, as the engineer/designer of this Distance class to make sure this is always true.
Separate Files
As is commonly done when designing a class, you are to separate your class from the program that will use the class. You will need the following 3 files:
main. cpp: contains the main function and any other global functions used to test your Distance class.
Distance. h: the Distance class header (aka interface) file. Contains the declaration of the Distance class.
Distance. cpp: the Distance class implementation file. Contains the implementations of all Distance member functions.
You will submit all 3 files, main. cpp, Distance. h and Distance. cpp. Here is the Distance. h file you MUST submit:
DO NOT CHANGE THIS FILE IN ANY WAY
// Distance. h file
#include
using namespace std;
class Distance
{
private:
unsigned feet;
double inches;
public:
/* Constructs a default Distance of 0 (0 feet and 0.0 inches)
*/
Distance();
/* Constructs a distance of ft feet and in inches,
unless in >= 12, in which case the values of feet and inches
are adjusted accordingly. A Distance will always be positive.
*/
Distance(unsigned ft, double in);
/* Constructs a distance of 0 ft and in inches,
unless in >= 12, in which case the values of feet and inches
are adjusted accordingly. A Distance will always be positive.
*/
Distance(double in);
/* Returns just the feet portion of the Distance
*/
unsigned getFeet() const;
/* Returns just the inches portion of the Distance
*/
double getInches() const;
/* Returns the entire distance as the equivalent amount of inches.
(e. g., 4 feet 3.5 inches would be returned as 51.5 inches)
*/
double distanceInInches() const;
/* Returns the entire distance as the equivalent amount of feet.
(e. g., 3 feet 6 inches would be returned as 3.5 feet)
*/
double distanceInFeet() const;
/* Returns the entire distance as the equivalent amount of meters.
1 inch equals 0.0254 meters.
(e. g., 2 feet 8.12 inches would be returned as 0.815848 meters)
*/
double distanceInMeters() const;
/* Returns the sum of 2 Distances.
*/
const Distance operator+(const Distance &rhs) const;
/* Returns the difference between 2 Distances.
*/
const Distance operator-(const Distance &rhs) const;
/* Outputs to the stream out the Distance in the format:
feet' inches'' (i. e. 3' 3.41'')
*/
friend ostream & operator<<(ostream &out, const Distance &rhs);
private:
/* Used by the 2 parameterized constructors to convert any negative values to positive and
inches >= 12 to the appropriate number of feet and inches.
*/
void init();
};
Use this main. cpp file for submission. You will want to add your own unit tests of the other public functions, but do not add them to the main. cpp file you are submitting.
int main()
{
Distance d1;
cout << "d1: " << d1 << endl;
Distance d2 = Distance(2, 5.9);
Distance d3 = Distance(3.75);
cout << "d2: " << d2 << endl;
cout << "d3: " << d3 << endl;
//test init helper function
Distance d4 = Distance(5, 19.34);
Distance d5 = Distance(100);
cout << "d4: " << d4 << endl;
cout << "d5: " << d5 << endl;
//test add (<12 inches)
cout << "d4 + d5: " << (d4 + d5) << endl;
//test add (>12 inches)
cout << "d2 + d4: " << (d2 + d4) << endl;
//test sub (0 ft)
cout << "d3 - d1: " << (d3 - d1) << endl;
//test sub (0 ft, negative conversion)
cout << "d1 - d3: " << (d1 - d3) << endl;
//test sub (positive ft & inch)
cout << "d4 - d2: " << (d4 - d2) << endl;
//test sub (negative ft & inch)
cout << "d2 - d4: " << (d2 - d4) << endl;
//test sub (negative ft, positive inch)
cout << "d4 - d5: " << (d4 - d5) << endl;
//test sub (positive ft, negative inch)
cout << "d5 - d2: " << (d5 - d2) << endl;
return 0;
}
Given this main function, if your constructors and operator functions are working correctly, your output will look like:
d1: 0' 0"
d2: 2' 5.9"
d3: 0' 3.75"
d4: 6' 7.34"
d5: 8' 4"
d4 + d5: 14' 11.34"
d2 + d4: 9' 1.24"
d3 - d1: 0' 3.75"
d1 - d3: 0' 3.75"
d4 - d2: 4' 1.44"
d2 - d4: 4' 1.44"
d4 - d5: 1' 8.66"
d5 - d2: 5' 10.1"

Answers: 3
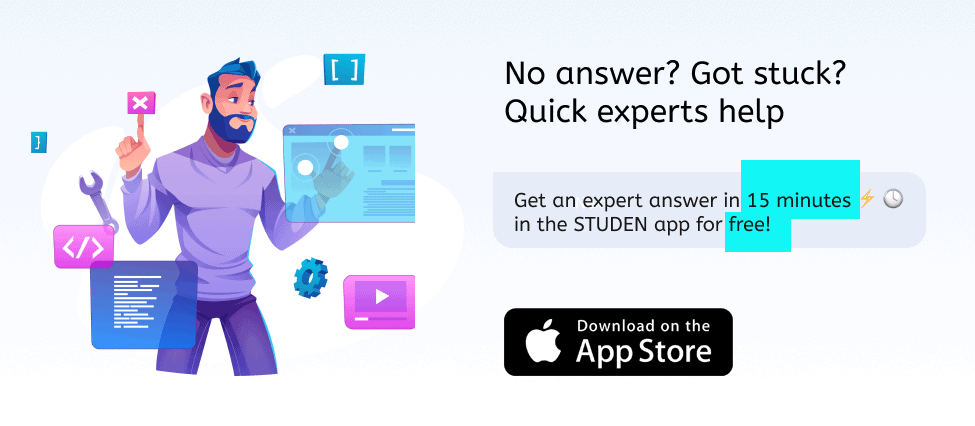
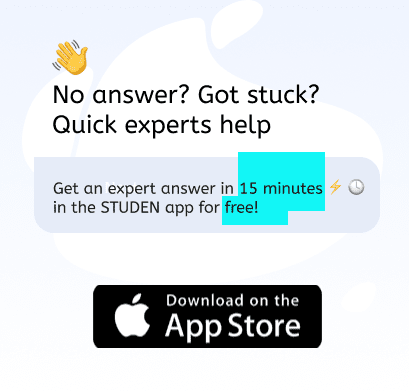
Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:00
What is added to the < meta > tag to describe the encoding type?
Answers: 1

Computers and Technology, 23.06.2019 11:30
The most accurate readings that you can take on an analog vom are when the meter's pointer is at the a. center scale. b. extreme right. c. near right. d. extreme left.
Answers: 1

Computers and Technology, 24.06.2019 01:10
Create a program that will take in a single x and y coordinate as the origin. after the input is provided, the output should be all of the coordinates (all 26 coordinates read from the “coordinates.json” file), in order of closest-to-farthest from the origin.
Answers: 1

Computers and Technology, 24.06.2019 07:00
Into what form does the barcode reader convert individual bar patterns?
Answers: 1
You know the right answer?
The Distance class will represent a distance in some number of feet and inches. This Distance object...
Questions
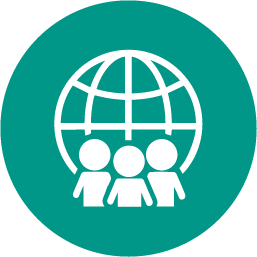
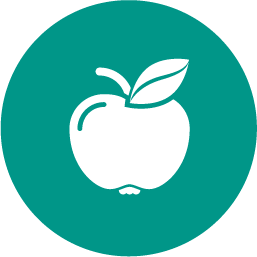





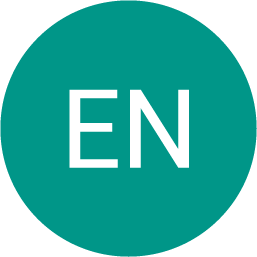





Mathematics, 10.03.2020 09:04



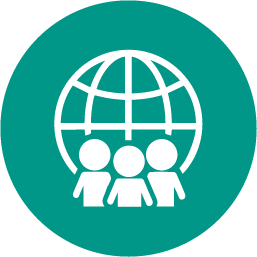

