
Computers and Technology, 17.10.2020 21:01 waterbug7571
Write the function for "fastest_words", which returns which words each player typed fastest. This function is called once both players have finished typing. It takes in a game as an argument. The game argument is a game data abstraction, like the one returned in Problem 9. You can access words in the game with selectors word_at, which takes in a game and the word_index (an integer). You can access the time it took any player to type any word using time. The fastest_words function returns a list of lists of words, one list for each player, and within each list the words they typed the fastest. In the case of a tie, consider the earliest player in the list (the smallest player index) to be the one who typed it the fastest. Be sure to use the accessor functions for the game data abstraction, rather than assuming a particular data format.
def fastest_words(game):
"""Return a list of lists of which words each player typed fastest.
Arguments:
game: a game data abstraction as returned by time_per_word.
Returns:
a list of lists containing which words each player typed fastest
"""
players = range(len(all_times(game))) # An index for each player
words = range(len(all_words(game))) # An index for each word
Here are some examples of it working correctly:
>>> from cats import game, fastest_words
>>> p0 = [2, 2, 3]
>>> p1 = [6, 1, 2]
>>> fastest_words(game(['What', 'great', 'luck'], [p0, p1]))
returns [['What'], ['great', 'luck']]
>>> p0 = [2, 2, 3]
>>> p1 = [6, 1, 3]
>>> fastest_words(game(['What', 'great', 'luck'], [p0, p1])) # with a tie, choose the first player
returns [['What','luck'], ['great']]
Below are the supplementary functions mentioned in the explanation
def game(words, times):
"""A data abstraction containing all words typed and their times."""
assert all([type(w) == str for w in words]), 'words should be a list of strings'
assert all([type(t) == list for t in times]), 'times should be a list of lists'
assert all([isinstance(i, (int, float)) for t in times for i in t]), 'times lists should contain numbers'
assert all([len(t) == len(words) for t in times]), 'There should be one word per time.'
return [words, times]
def word_at(game, word_index):
"""A selector function that gets the word with index word_index"""
assert 0 <= word_index < len(game[0]), "word_index out of range of words"
return game[0][word_index]
def all_words(game):
"""A selector function for all the words in the game"""
return game[0]
def all_times(game):
"""A selector function for all typing times for all players"""
return game[1]
def time(game, player_num, word_index):
"""A selector function for the time it took player_num to type the word at word_index"""
assert word_index < len(game[0]), "word_index out of range of words"
assert player_num < len(game[1]), "player_num out of range of players"
return game[1][player_num][word_index]
def game_string(game):
"""A helper function that takes in a game object and returns a string representation of it"""
return "game(%s, %s)" % (game[0], game[1])

Answers: 1
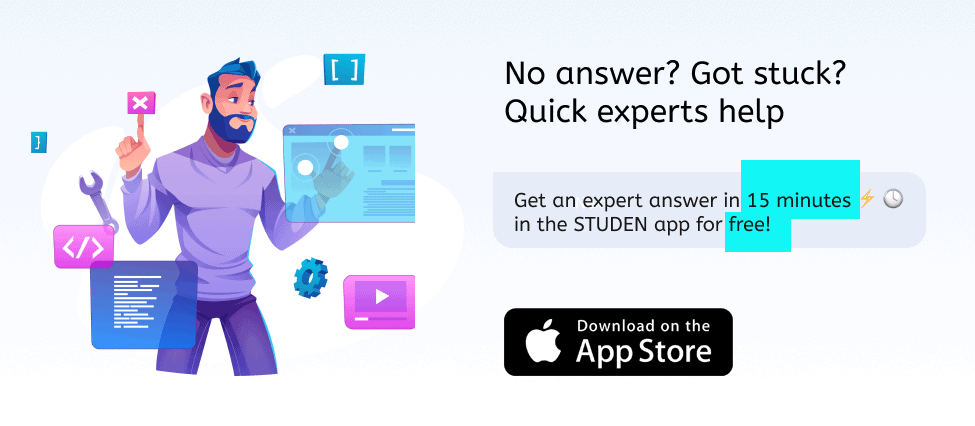
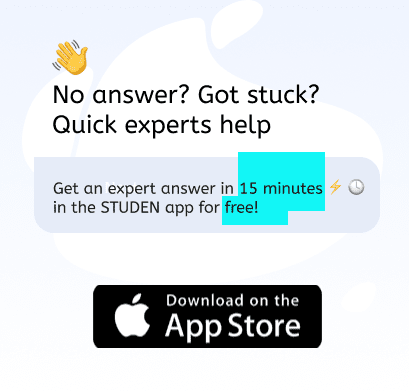
Another question on Computers and Technology

Computers and Technology, 21.06.2019 21:00
When a rectangular region is defined using an appropriate style, which value matches the specified edge of the clipping region to the edge of the parent element?
Answers: 3

Computers and Technology, 22.06.2019 11:10
Look at the far left lane in the picture. explain what the red car is doing and what it needs to do to travel safely.
Answers: 2

Computers and Technology, 22.06.2019 17:00
The two main ways in which marketers address the competition with their strategies are by satisfying a need better than a competition and by
Answers: 2

You know the right answer?
Write the function for "fastest_words", which returns which words each player typed fastest. This fu...
Questions
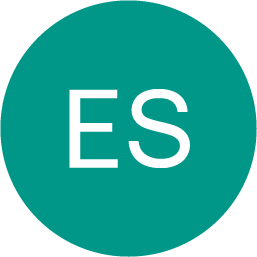
Spanish, 20.09.2020 04:01
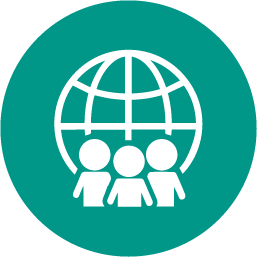


Mathematics, 20.09.2020 04:01

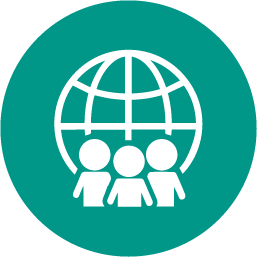
History, 20.09.2020 04:01
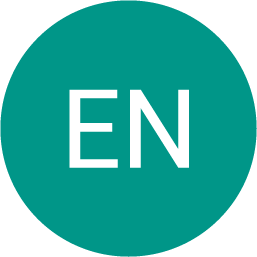

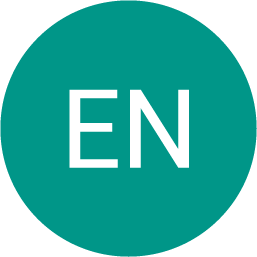

Mathematics, 20.09.2020 04:01


Mathematics, 20.09.2020 04:01
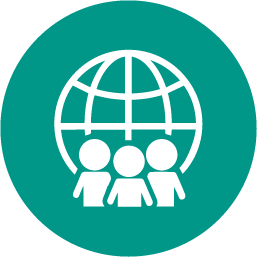
History, 20.09.2020 04:01

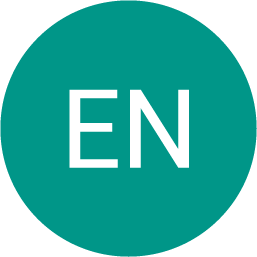

Mathematics, 20.09.2020 04:01
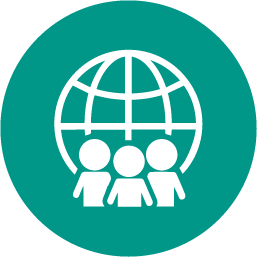
History, 20.09.2020 04:01

Mathematics, 20.09.2020 04:01
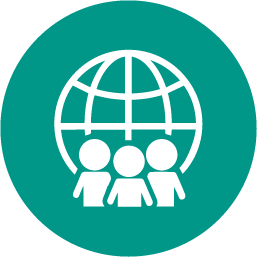