
Computers and Technology, 13.04.2021 01:00 ashleyvalles16
The goal of this lab is to write a code, Birthday. java, for conducting an experiment to test the Birthday Paradox, which states that if 23 people gather, the chances are 50-50 that there are two people in the group having the same birthdays. This observation comes from the following analysis. Assume that there are 365 days in a year and the birthdays of people are evenly distributed on the 365 possible dates. In other words, for each day of a year, the expected proportion of the people born on that day is 1/365 of the entire population. Under these assumptions, the probability that randomly selected 23 people have 23 distinct birthdays collectively is: 365 β 364 β ... β 343 36523 (1) The denominator represents the number of possible choices for the birthdays collectively given to the 23 people. The numerator represents the number of possibilities for the 23 people to select their birthdays so that no two people choose the same birthdays. There, the first person has 365 possibilities, the second has 364 because he/she must not pick the one that the first person has chosen, the third person has 363 because he/she must not pick the ones that the first and the second persons have chosen, and so on. The fraction has the value approximately equal to 0.50, so the chances are almost 50-50 that there are two people having the same birthdays. The program The centerpiece of the code is a method, oneTrial, that, given a number of people as its parameter, randomly selects birthdays for those people and produces for each day how many people have selected that day as their birthdays. More formally, the method receives the number of people, nPeople, as its formal parameter and returns an array of int whose length is 365. The method first instantiates an array of 365 elements, named theCounts. Then, it enters a for-loop that iterates 1, ..., nPeople with some iteration variable. In each iteration of the loop, the method selects a random integer between 0 and 364, and then increases the element of the array theCounts at the selected position by 1. After quitting the loop, the method returns the array theCounts. Since the elements of arrays of numbers are initialized with the value 0, explicit initialization is not needed. After receiving the return value of oneTrial, we examine the elements of the array, looking for an element with the value greater than or equal to 2. Such an element indicates the existence of multiple people having the same birthdays. We write a method, hasAHit, for executing this search. The method receives an int array as its parameter and returns a boolean. Using a for-loop, the method scans the elements of the array given as the formal parameter. On encountering an element whose value is greater than or equal to 2, the method immediately returns true, ignoring the remaining elements of the array. If this does not happen, the loop terminates. At that point, the method returns false. 1 Using the above two methods, we write another method, experiment1. This method has two formal parameters. The first is an int named nPeople. This is the value to be passed to oneTrial as the number of people. The second is an int as well and is named nReps. This is the number of times to execute oneTrial. Use a for-loop to count the executions of oneTrial. After each execution, feed the return array of oneTrial to hasAHit. If hasAHit returns true, increase the value of a double variable, hitRate, by 1. The initial value of hitRate is 0. After completing the loop, we divide hitRate by nReps. The value of hitRate then becomes the proportion of the repetitions in which the random birthday selections generated multiple people having the same birthdays. The method experiment1 reports this ratio before terminating. The main method receives the quantities for nPeople and nReps and calls experiment1 with these two values as the actual parameters. To print the average, use "%.3f" in printf so that exactly three digits appear after the decimal point.
output example:
% java Birthday
Enter the no. of people: 23
Enter the no. of repetitions: 100
Experiment 1 Probability Of Hits: 0.490
Experiment 2
Average No. of 0s: 342.760
Average No. of 1s: 21.500
Average No. of 2s: 0.720
Average No. of >=3s: 0.020

Answers: 1
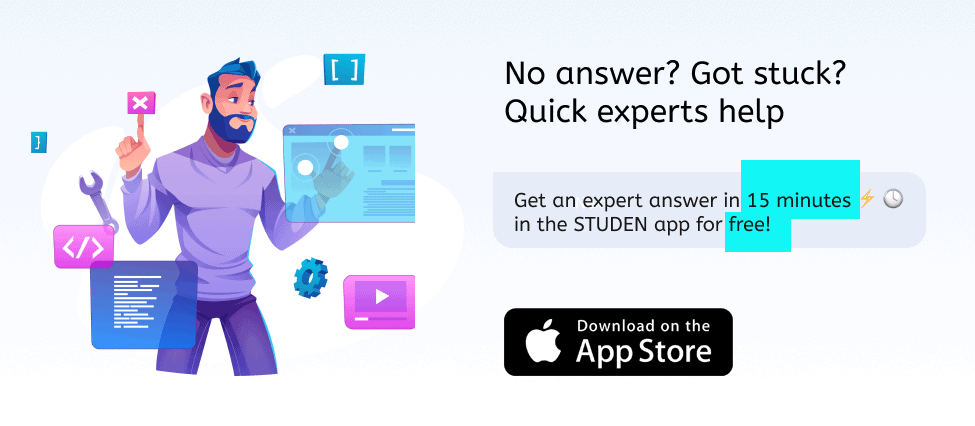
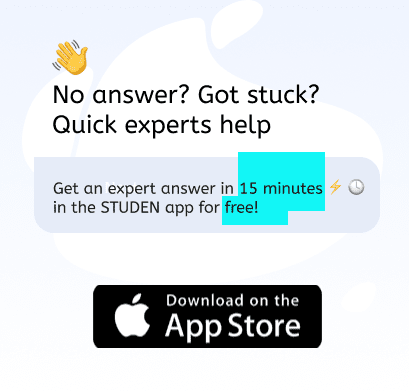
Another question on Computers and Technology

Computers and Technology, 21.06.2019 14:00
Var add = function( x, y ) { return ( x + y ); } alert( add (5, 3) ); 11. (refer to code example 2.) the function a. accepts 2 parameters and returns 2 values. b. accepts 2 parameters and returns 1 value. c. accepts 2 parameters and does not return a value. d. does not accept a parameter and returns 1 value.
Answers: 1

Computers and Technology, 22.06.2019 09:40
In the lab, which of the following displayed a list of all installed services and included a description of the service, the current state, and whether the service started automatically or manually? a. the services manager b. the applications summary c. the recommended services d. list the safe services list
Answers: 2

Computers and Technology, 22.06.2019 19:20
1)consider the following code snippet: #ifndef book_h#define book_hconst double max_cost = 1000.0; class book{public: book(); book(double new_cost); void set_cost(double new_cost); double get_cost() const; private: double cost; }; double calculate_terms(book bk); #endifwhich of the following is correct? a)the header file is correct as given.b)the definition of max_cost should be removed since header files should not contain constants.c)the definition of book should be removed since header files should not contain class definitions.d)the body of the calculate_terms function should be added to the header file.
Answers: 1

Computers and Technology, 23.06.2019 04:31
Jennifer has to set up a network in a factory with an environment that has a lot of electrical interference. which cable would she prefer to use? jennifer would prefer to use because its metal sheath reduces interference.
Answers: 1
You know the right answer?
The goal of this lab is to write a code, Birthday. java, for conducting an experiment to test the Bi...
Questions
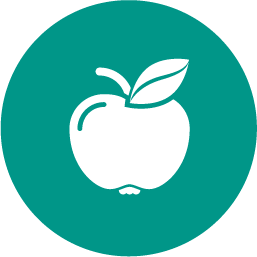


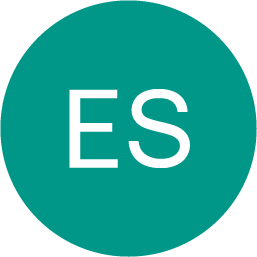
Spanish, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00

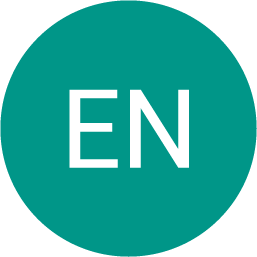


Mathematics, 04.11.2020 21:00

Arts, 04.11.2020 21:00
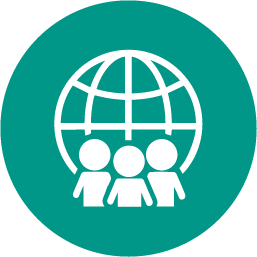
Social Studies, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00
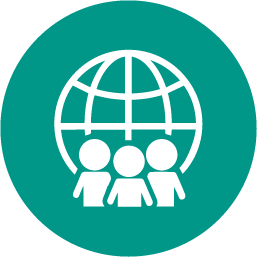
History, 04.11.2020 21:00
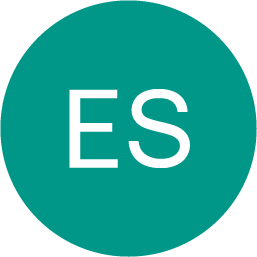

Chemistry, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00
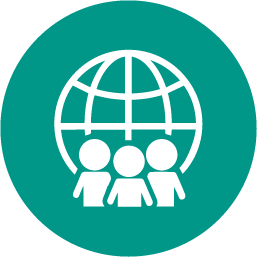
History, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00

Mathematics, 04.11.2020 21:00