
Computers and Technology, 24.05.2021 15:20 Kurlyash
The program below represents decimal integers as a linked list of digits. It asks for 2 positive integers, stores them as linked lists, computes the sum, and outputs it. It then freeO's all nodes of all 3 lists. a. You must use my struct Digi tNode b. I strongly suggest that you represent the integers in little-endian order. That will make add 0) way easier. Please write: obtainPostiveInt 。numberList () o add printList o freeList Sample output: (No surprises here, it is just decimal integer addition, but these are some interesting boundary cases to make sure you handle.)
C CODE (COPY/PASTE):
/**
*--- ---*
*--- byDigitAdder. c ---*
*--- ---*
*--- This file defines a C program that represents positive ---*
*--- decimal integers as linked lists of digits. It asks the user ---*
*--- for 2 integers, computes the sum, and outputs it. ---*
*--- ---*
*-*
*--- ---*
*--- Version 1a 2018 February 11 Joseph Phillips ---*
*--- ---*
**/
#include
#include
#include
#define NUM_TEXT_LEN 256// PURPOSE: To represent one digit of a decimal number.
struct DigitNode
{
int digit_; // I suggest you range this in [0..9]
struct DigitNode* nextPtr_; // I suggest you make this point to
// the next most significant digit.
};
// PURPOSE: To obtain the text of a decimal integer into array 'numberCPtr'
// of length 'numberTextLen'. 'descriptionCPtr' is printed because it
// tells the user the integer that is expected. Ending '\n' from
// 'fgets()' is replaced with '\0'. No return value.
void obtainPostiveInt(char* numberCPtr,
int numberTextLen,
const char* descriptionCPtr
)
{
// YOUR CODE HERE
}
// PURPOSE: To build and return a linked list IN LITTLE ENDIAN ORDER
// of the decimal number whose text is pointed to by 'numberCPtr'.
// If 'numberCPtr' points to the string "123" then the linked list
// returned is 'digit_=3' -> 'digit_=2' -> 'digit_=1' -> NULL.
struct DigitNode*
numberList (const char* numberCPtr
)
{
// YOUR CODE HERE
}
// PURPOSE: To build and return a linked list IN LITTLE ENDIAN ORDER
// of the decimal number that results from adding the decimal numbers
// whose digits are pointed to by 'list0' and 'list1'.
struct DigitNode*
add (const struct DigitNode* list0,
const struct DigitNode* list1
)
{
// YOUR CODE HERE
}
// PURPOSE: To print the decimal number whose digits are pointed to by 'list'.
// Note that the digits are IN LITTLE ENDIAN ORDER. No return value.
void printList (const struct DigitNode* list
)
{
// YOUR CODE HERE
}
// PURPOSE: To print the nodes of 'list'. No return value.
void freeList (struct DigitNode* list
)
{
// YOUR CODE HERE
}
// PURPOSE: To coordinate the running of the program. Ignores command line
// arguments. Returns 'EXIT_SUCCESS' to OS.
int main ()
{
char numberText0[NUM_TEXT_LEN];
char numberText1[NUM_TEXT_LEN];
struct DigitNode* operand0List = NULL;
struct DigitNode* operand1List = NULL;
struct DigitNode* sumList = NULL;
obtainPostiveInt(numberText0,NUM_TE XT_LEN,"first");
obtainPostiveInt(numberText1,NUM_TE XT_LEN,"second");
operand0List = numberList(numberText0);
operand1List = numberList(numberText1);
sumList = add(operand0List, operand1List);
printList(operand0List);
printf(" + ");
printList(operand1List);
printf(" = ");
printList(sumList);
printf("\n");
freeList(sumList);
freeList(operand1List);
freeList(operand0List);
return(EXIT_SUCCESS);
}

Answers: 1
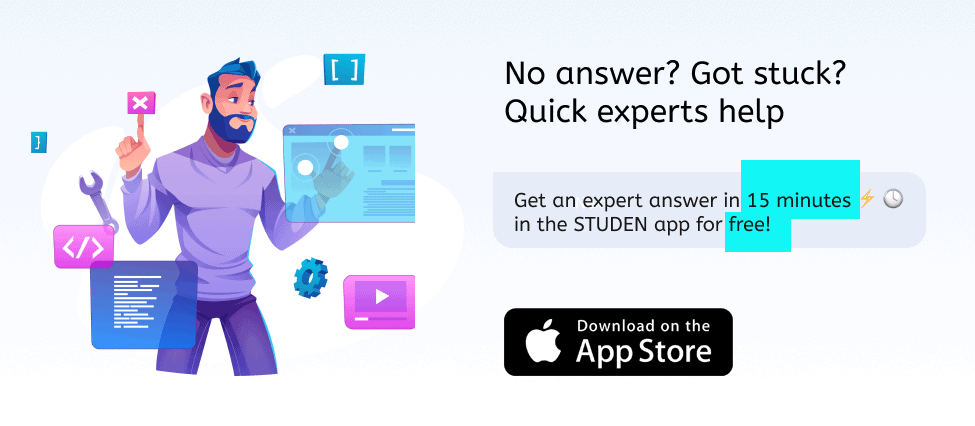
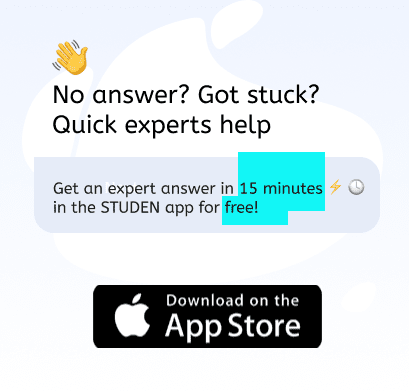
Another question on Computers and Technology

Computers and Technology, 22.06.2019 03:20
Which of these is a benefit of social networking? oa. hiding your true identity from friendsob. avoiding talking to people in personoc. spending time with friends instead of studyingod. connecting with new people
Answers: 2

Computers and Technology, 23.06.2019 02:00
What is the main benefit of minimizing the ribbon in word? more options will be accessible through customized keystrokes. more of the document will be viewable without needing to scroll. fewer controls will be accessible to the user by using the mouse. fewer editing options will be available without entering a password.
Answers: 1

Computers and Technology, 23.06.2019 03:30
Many everyday occurrences can be represented as a binary bit. for example, a door is open or closed, the stove is on or off, and the fog is asleep or awake. could relationships be represented as a binary value? give example.
Answers: 1

Computers and Technology, 23.06.2019 10:00
Install and use wireshark program ( send back screen shots and other vital information) case project 3-2: decode a tcp segment in a wireshark capture in this chapter, you walked through tcp segment to interpret the data included in its header. in this project, you use wireshark to capture your own http messafes, examine the tcp headers, and practice interpreting the data you'll find there. 1. open wireshark and snap the window to one side of your screen. open a browser and snap that window to the other side of your screen so you can see both windows.
Answers: 2
You know the right answer?
The program below represents decimal integers as a linked list of digits. It asks for 2 positive int...
Questions

Arts, 16.11.2019 17:31
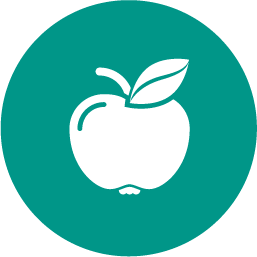
Physics, 16.11.2019 17:31
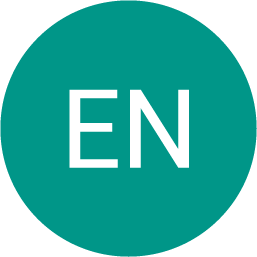


Chemistry, 16.11.2019 17:31


Mathematics, 16.11.2019 17:31

Mathematics, 16.11.2019 17:31
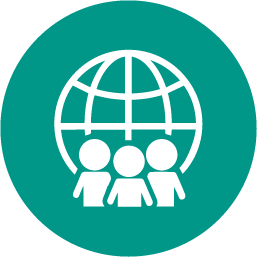



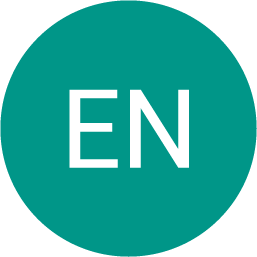
English, 16.11.2019 17:31

Mathematics, 16.11.2019 17:31
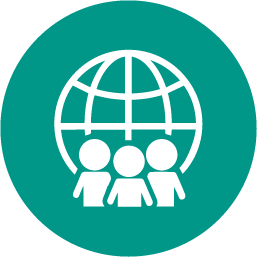
History, 16.11.2019 17:31

Mathematics, 16.11.2019 17:31
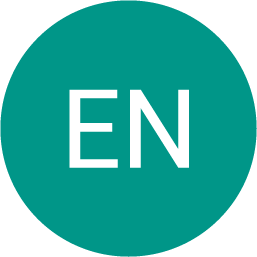


Mathematics, 16.11.2019 17:31
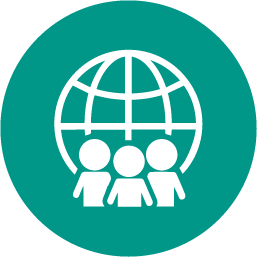
History, 16.11.2019 17:31