
Computers and Technology, 24.02.2022 04:10 angelisabeast5430
Using Python:
Step 1: Prompt the user to enter a string of their choosing. Store the text in a string. Output the string.
Step 2: Implement the print_menu() function to print the following command menu.
Sample output:
MENU
c - Number of non-whitespace characters
w - Number of words
f - Fix capitalization
r - Replace punctuation
s - Shorten spaces
q - Quit
Step 3: Implement the execute_menu() function that takes 2 parameters: a character representing the user's choice and the user provided sample text. execute_menu() performs the menu options, according to the user's choice, by calling the appropriate functions described below.
Step 4:
In the main program, call print_menu() and prompt for the user's choice of menu options for analyzing/editing the string. Each option is represented by a single character.
If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling execute_menu(). Then, print the menu and prompt for a new option. Continue until the user enters 'q'.
Step 5: Implement the get_num_of_non_WS_characters() function. get_num_of_non_WS_characters() has a string parameter and returns the number of characters in the string, excluding all whitespace. Call get_num_of_non_WS_characters() in the execute_menu() function, and then output the returned value.
Sample output with steps 1-5:
Enter a sample text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
You entered: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
MENU
c - Number of non-whitespace characters
w - Number of words
f - Fix capitalization
r - Replace punctuation
s - Shorten spaces
q - Quit
Choose an option: c
Number of non-whitespace characters: 181
Step 6: Implement the get_num_of_words() function. get_num_of_words() has a string parameter and returns the number of words in the string. Hint: Words end when a space is reached except for the last word in a sentence. Call get_num_of_words() in the execute_menu() function, and then output the returned value.
Step 7: Implement the fix_capitalization() function. fix_capitalization() has a string parameter and returns an updated string, where lowercase letters at the beginning of sentences are replaced with uppercase letters. fix_capitalization() also returns the number of letters that have been capitalized. Call fix_capitalization() in the execute_menu() function, and then output the number of letters capitalized followed by the edited string.
Sample Output:
Number of letters capitalized: 3
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!
Step 8: Implement the replace_punctuation() function. replace_punctuation() has a string parameter and two keyword argument parameters exclamation_count and semicolon_count. replace_punctuation() updates the string by replacing each exclamation point (!) character with a period (.) and each semicolon (;) character with a comma (,). replace_punctuation() also counts the number of times each character is replaced and outputs those counts. Lastly, replace_punctuation() returns the updated string. Call replace_punctuation() in the execute_menu() function, and then output the edited string.
Sample Output:
Punctuation replaced e
xclamation_count: 1
semicolon_count: 2
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here, our hopes and our journeys continue.
Step 9: Implement the shorten_space() function. shorten_space() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with a single space. shorten_space() returns the string. Call shorten_space() in the execute_menu() function, and then output the edited string.
Sample Output:
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!

Answers: 2
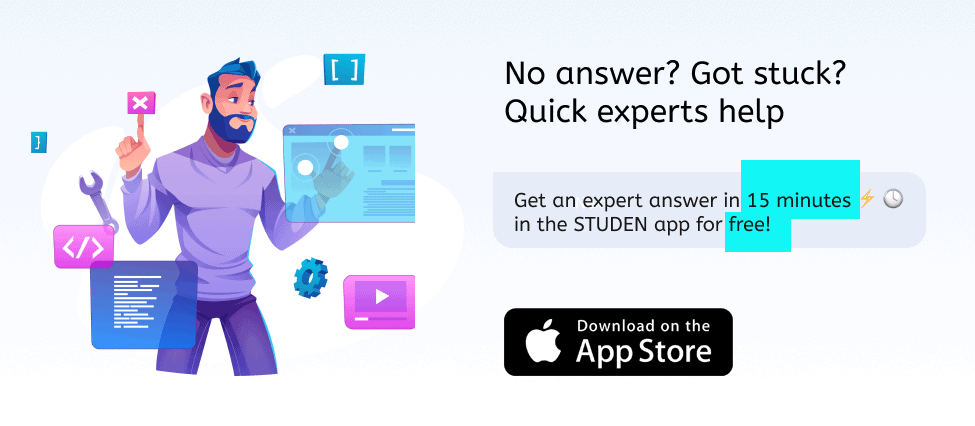
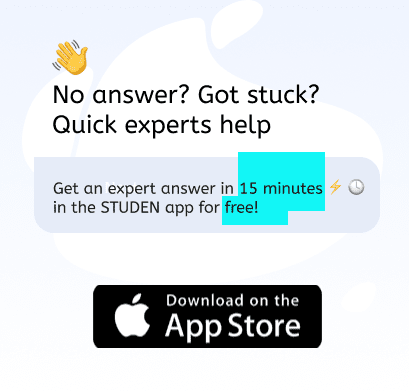
Another question on Computers and Technology

Computers and Technology, 21.06.2019 18:00
What ordering of tcp flags makes up the three-way handshake?
Answers: 2

Computers and Technology, 22.06.2019 11:00
When building customer relationships through email what should you not do? question 2 options: utilize proper grammar, spelling, and punctuation type in all capital letters use hyperlinks rather than attachments respond to all emails within 24 hours
Answers: 1

Computers and Technology, 22.06.2019 17:30
Under which key category do the page up and page down keys fall? page up and page down keys fall under the keys category.
Answers: 1

Computers and Technology, 22.06.2019 23:30
To check spelling errors in a document, the word application uses the to determine appropriate spelling. internet built-in dictionary user-defined words other text in the document
Answers: 1
You know the right answer?
Using Python:
Step 1: Prompt the user to enter a string of their choosing. Store the text in a str...
Questions
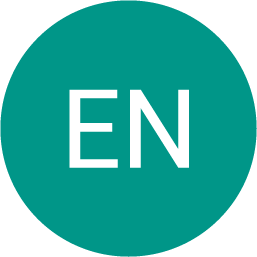
English, 06.11.2020 01:00

SAT, 06.11.2020 01:00

Mathematics, 06.11.2020 01:00
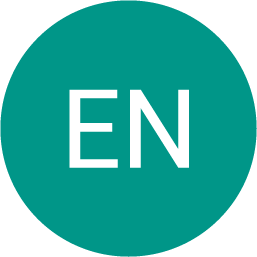


Mathematics, 06.11.2020 01:00


Mathematics, 06.11.2020 01:00

Computers and Technology, 06.11.2020 01:00

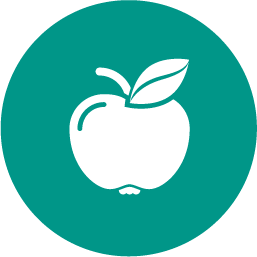
Physics, 06.11.2020 01:00

Chemistry, 06.11.2020 01:00

Mathematics, 06.11.2020 01:00


Mathematics, 06.11.2020 01:00
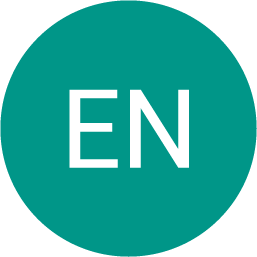

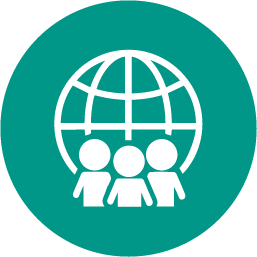

Mathematics, 06.11.2020 01:00